Web Programming with Spring MVC and Hibernate
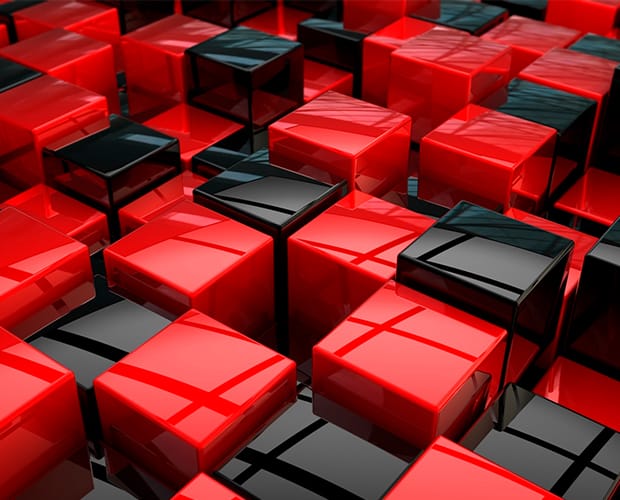
Get ready for your exam by enrolling in our comprehensive training course. This course includes a full set of instructional videos designed to equip you with in-depth knowledge essential for passing the certification exam with flying colors.
$14.99 / $24.99
Getting Started
-
1. What is Spring?7m
-
2. Setting Up Your System16m
-
3. Introducing Maven14m
-
4. Using Maven on the Command Line9m
-
5. Spring "Hello World"21m
-
6. Class Path Contexts5m
0 / 12|1hr 24min
-
1. Constructor Arguments8m
-
2. Setting Bean Properties7m
-
3. Dependency Injection12m
-
4. Bean Scope4m
-
5. Init and Destroy Methods9m
-
6. Factory Beans and Methods13m
-
7. The P Namespace6m
-
8. Setting List Properties6m
-
9. Lists of Beans5m
-
10. Inner Beans4m
-
11. Property Maps4m
-
12. Arbitrary Maps as Bean Properties6m
Autowiring
-
1. Autowiring by Type9m
-
2. Autowiring by Name6m
-
3. Autowiring by Constructor4m
-
4. Default Autowiring7m
-
5. Removing Autowire Ambiguities5m
Wiring with Annotations
-
1. Adding Support for Annotation-Based Wiring6m
-
2. The "Autowired" Annotation8m
-
3. Optional Beans3m
-
4. Using Qualifiers8m
-
5. The Resource Annotation (JSR-250)4m
-
6. Annotation-Based Init and Destroy Methods2m
-
7. The Inject annotation (JSR-330)5m
-
8. Automatic Bean Discovery5m
-
9. Setting Property Values via Annotations4m
Spring Expression Language (SPEL)
-
1. Introducing SPEL13m
-
2. Using SPEL with Annotations5m
-
3. Some useful SPEL Operators7m
Working with Databases
-
1. Creating a Database with MySQL15m
-
2. Using Property Files9m
-
3. Implementing the DAO Pattern4m
-
4. Downloading a Connector Jar3m
-
5. Configuring Connection Pooling with Apache DBCP12m
-
6. JDBC Templates5m
-
7. Querying the Database12m
-
8. Database Exceptions6m
-
9. Named Parameters10m
-
10. Update Statements5m
-
11. Getting Placeholder Values from Beans8m
-
12. Adding an Update Method to the DAO7m
-
13. Batch Updates: Prepared Statements12m
-
14. Transactions12m
Web Application Basics with Spring MVC
-
1. A Basic Non-Spring Web App8m
-
2. Bringing in Maven6m
-
3. The Dispatcher Servlet12m
-
4. Adding a Controller7m
-
5. View Resolvers9m
-
6. Adding Data to the Session8m
-
7. Using Spring Data Models12m
-
8. Using JSTL (JSP Standard Tag Library)10m
-
9. Configuring a JNDI Data Source21m
-
10. Bringing in the DAO Code7m
-
11. Loading Bean Containers with ContextLoaderListener9m
-
12. Creating a Datasource Bean6m
-
13. Adding a Service Layer15m
-
14. Adding a New Controller11m
-
15. Getting URL Parameters6m
Working with Web Forms
-
1. Creating a Form11m
-
2. Getting Form Values6m
-
3. Adding CSS Styles10m
-
4. Serving Static Resources10m
-
5. Adding Hibernate Form Validation Support13m
-
6. More Form Validation Tags8m
-
7. Making Forms Remember Values9m
-
8. Displaying Form Validation Errors7m
-
9. Creating a Custom Validation Annotation19m
-
10. Hooking Up the Controller and Database Code10m
-
11. Exception Handling in Spring MVC13m
Aspect-Oriented Programming (AOP)
-
1. A Base Project for Working with Aspects9m
-
2. A Simple Aspect Example13m
-
3. Annotation-Based Aspects13m
-
4. Wildcards in Pointcut Expressions15m
-
5. Advice Types: After, Around and Others11m
-
6. Proxies, Interfaces and Aspects13m
-
7. The “Within” Pointcut Designator4m
-
8. “This”, “Target” and Matching Subpackages8m
-
9. Annotation-Specific PCDs13m
-
10. The “Bean” PCD3m
-
11. The “Args” PCD8m
-
12. Getting Target Method Arguments3m
-
13. Getting Arguments Using “Args”7m
-
14. Combining Pointcuts6m
-
15. Introductions: Adding Functionality Using Aspects11m
Spring Security and Managing Users
-
1. Servlets Filters: A Review9m
-
2. Adding a Spring Security Filter10m
-
3. Adding a Spring Login Form17m
-
4. Serving Static Resources: Access Rules3m
-
5. Customising the Login Form10m
-
6. Displaying Login Errors9m
-
7. Authorising Users from a Database14m
-
8. Adding a “Create Account” Form16m
-
9. Making the “Create Account” Form Work18m
-
10. Adding Validation to the User Form18m
-
11. Dealing with Duplicate Usernames14m
-
12. Storing Validation Messages in a Property File10m
-
13. Using JQuery to verify the password28m
-
14. Using Property File Values in JSPs7m
-
15. Adding a Logout Link6m
-
16. Working With Roles9m
-
17. Outputting Text Based on Authentication Status6m
-
18. Row Mapping with BeanPropertyRowMapper11m
-
19. Using Custom Authentication Queries: Case Sensitive Usernames6m
-
20. Method-Level Access Control11m
-
21. Catching Secure Annotation Violations13m
-
22. Adding “Remember Me” Functionality10m
-
23. Encrypting Passwords20m
Apache Tiles and Spring MVC
-
1. Tiles Dependencies11m
-
2. “Hello World” Apache Tiles13m
-
3. Adding Headers and Footers11m
-
4. Formatting the Offers Application10m
-
5. Creating Tiles from JSP Files13m
Logging and Testing
-
1. Adding Log4J Logging9m
-
2. Resolving Logging Conflicts8m
-
3. Using Logging5m
-
4. Creating a MySQL Test Database4m
-
5. Using Spring Profiles4m
-
6. Creating JUnit Tests14m
-
7. Coding the JUnit DAO Tests15m
Improving the "Offers" Web Application
-
1. Normalizing the Database9m
-
2. Querying Tables with Foreign Keys and Refactoring the DAO Layer20m
-
3. Refactoring the Web Layer9m
-
4. Getting the Username of the Logged-In User6m
-
5. Deleting from Tables with Foreign Keys and a Little Bugfix3m
-
6. Custom RowMappers7m
-
7. Conditional Database-Dependent Text in JSPs11m
-
8. Editing Database Objects with Forms12m
-
9. Multiple Form Submits and Optional Parameters14m
-
10. Adding a Confirm Dialog with JQuery8m
Hibernate
-
1. Introducing Hibernate6m
-
2. A Simple Hibernate Query23m
-
3. Saving Objects8m
-
4. Validation Groups and Password Encryption18m
-
5. Translating Hibernate Exceptions to Spring Exceptions6m
-
6. Queries with Criteria10m
-
7. Mapping Many-to-One Relationships16m
-
8. Restrictions on Joined Tables4m
-
9. Multiple Criteria8m
-
10. Deleting Objects8m
-
11. Completing the Offers DAO10m
Spring Webflow
-
1. Introducing Webflow4m
-
2. Creating a Flow Registry10m
-
3. Hooking Up URLs to Webflows9m
-
4. Connecting Webflow and Apache Tiles10m
-
5. Creating a “Messages” Table6m
-
6. Creating a “Message” Class13m
-
7. Adding a Message Form14m
-
8. Transitions6m
-
9. Action States10m
-
10. Linking to Webflows8m
-
11. Validating Webflow Forms10m
-
12. Accessing User Details in Webflow14m
JSON and AJAX
-
1. Creating a JSON Server25m
-
2. Updating Dynamically with jQuery32m
-
3. Generating Pages with Javascript27m
-
4. Adding Reply Boxes13m
-
5. Showing and Hiding the Reply Forms12m
-
6. Stopping and Starting the Timer3m
-
7. Getting the Text from the Right TextArea8m
-
8. Posting Back JSON Data18m
-
9. Giving the User Feedback12m
-
10. Sending Email with Springmail8m
Outro
-
1. Some Final Words ...2m