Understanding Weird Parts of JavaScript
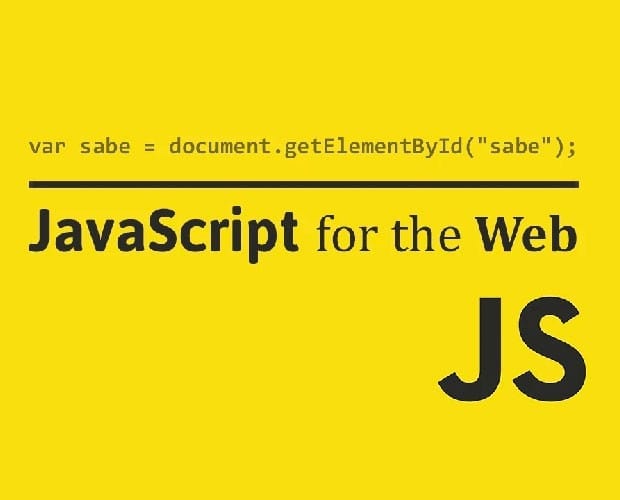
Get ready for your exam by enrolling in our comprehensive training course. This course includes a full set of instructional videos designed to equip you with in-depth knowledge essential for passing the certification exam with flying colors.
$14.99 / $24.99
Getting Started
-
1. Introduction and The Goal of This Course4m 56s
-
2. Setup3m 27s
-
3. Big Words and Javascript1m 25s
-
4. Understanding, Frameworks, and The Weird Parts4m 17s
Execution Contexts and Lexical Environments
-
1. Conceptual Aside: Syntax Parsers, Execution Contexts, and Lexical Environments7m 35s
-
2. Conceptual Aside: Name/Value Pairs and Objects4m 8s
-
3. The Global Environment and The Global Object10m 58s
-
4. The Execution Context - Creation and Hoisting9m 43s
-
5. Conceptual Aside: Javascript and 'undefined'8m 4s
-
6. The Execution Context - Code Execution2m 54s
-
7. Conceptual Aside: Single Threaded, Synchronous Execution2m 16s
-
8. Function Invocation and the Execution Stack8m 6s
-
9. Functions, Context, and Variable Environments7m 56s
-
10. The Scope Chain17m 25s
-
11. Scope, ES6, and let4m 18s
-
12. What About Asynchronous Callbacks?10m 26s
Types and Operators
-
1. Conceptual Aside: Types and Javascript2m 50s
-
2. Primitive Types5m 17s
-
3. Conceptual Aside: Operators6m 32s
-
4. Operator Precedence and Associativity14m 14s
-
5. Conceptual Aside: Coercion6m 14s
-
6. Comparison Operators19m 42s
-
7. Existence and Booleans7m 24s
-
8. Default Values7m 51s
-
9. Framework Aside: Default Values7m 11s
Objects and Functions
-
1. Objects and the Dot15m 23s
-
2. Objects and Object Literals10m 32s
-
3. Framework Aside: Faking Namespaces8m 7s
-
4. JSON and Object Literals7m 17s
-
5. Functions are Objects8m 3s
-
6. Function Statements and Function Expressions20m 32s
-
7. Conceptual Aside: By Value vs By Reference16m 9s
-
8. Objects, Functions, and 'this'21m 26s
-
9. Conceptual Aside: Arrays - Collections of Anything5m 39s
-
10. 'arguments' and spread12m
-
11. Framework Aside: Function Overloading4m 43s
-
12. Conceptual Aside: Syntax Parsers2m 25s
-
13. Dangerous Aside: Automatic Semicolon Insertion5m 47s
-
14. Framework Aside: Whitespace4m 25s
-
15. Immediately Invoked Functions Expressions (IIFEs)17m 7s
-
16. Framework Aside: IIFEs and Safe Code8m 5s
-
17. Understanding Closures11m 9s
-
18. Understanding Closures - Part 219m 20s
-
19. Framework Aside: Function Factories12m 24s
-
20. Closures and Callbacks8m 26s
-
21. call(), apply(), and bind()20m 54s
-
22. Functional Programming20m 17s
-
23. Functional Programming - Part 28m 5s
Object-Oriented Javascript and Prototypal Inheritance
-
1. Conceptual Aside: Classical vs Prototypal Inheritance5m 11s
-
2. Understanding the Prototype14m 2s
-
3. Everything is an Object (or a primitive)5m 46s
-
4. Reflection and Extend14m 59s
Building Objects
-
1. Function Constructors, 'new', and the History of Javascript15m 53s
-
2. Function Constructors and '.prototype'10m 24s
-
3. Dangerous Aside: 'new' and functions4m 16s
-
4. Conceptual Aside: Built-In Function Constructors10m 32s
-
5. Dangerous Aside: Built-In Function Constructors3m 57s
-
6. Dangerous Aside: Arrays and for..in3m 16s
-
7. Object.create and Pure Prototypal Inheritance12m 37s
-
8. ES6 and Classes6m 27s
Odds and Ends
-
1. Initialization5m 41s
-
2. 'typeof' , 'instanceof', and Figuring Out What Something Is6m 41s
-
3. Strict Mode5m 51s
Examining Famous Frameworks and Libraries
-
1. Learning From Other's Good Code3m 51s
-
2. Deep Dive into Source Code: jQuery - Part 121m 31s
-
3. Deep Dive into Source Code: jQuery - Part 215m 38s
-
4. Deep Dive into Source Code: jQuery - Part 311m 22s
Let's Build a Framework / Library!
-
1. Requirements2m 58s
-
2. Structuring Safe Code3m 7s
-
3. Our Object and Its Prototype9m 17s
-
4. Properties and Chainable Methods16m 58s
-
5. Adding jQuery Support5m 22s
-
6. Good Commenting2m 21s
-
7. Let's Use Our Framework8m 11s
BONUS Lectures
-
1. TypeScript, ES6, and Transpiled Languages4m 33s
BONUS: Getting Ready for ECMAScript 6
-
1. Existing and Upcoming Features54s