TypeScript Complete Course
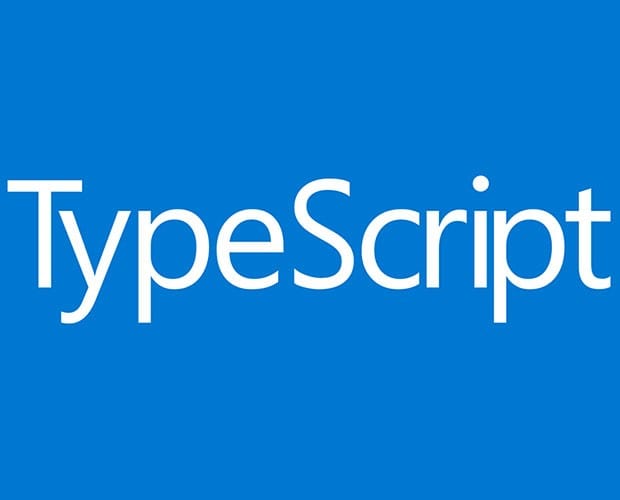
Get ready for your exam by enrolling in our comprehensive training course. This course includes a full set of instructional videos designed to equip you with in-depth knowledge essential for passing the certification exam with flying colors.
$14.99 / $24.99
Getting Started
-
1. What is TypeScript?3m 22s
-
2. Why TypeScript and How to use it?3m 47s
-
3. Installing TypeScript2m 12s
-
4. Using TypeScript2m 44s
-
5. Setting up the Course Workspace6m 14s
Using Types for a better Code
-
1. Type Basics2m 19s
-
2. Numbers & Booleans1m 40s
-
3. Assigning Types Explicitly2m 7s
-
4. Arrays and Types3m 3s
-
5. Tuples1m 55s
-
6. Enums3m 38s
-
7. The "Any" Type1m 41s
-
8. Understanding the created JavaScript Code1m 38s
-
9. Using Types in Functions (Arguments & Return Values)4m 31s
-
10. Functions as Types3m 59s
-
11. Objects and Types4m 44s
-
12. Example: Putting it all together in a Complex Object1m 28s
-
13. Creating custom Types with Type Aliases2m 29s
-
14. Allowing multiple Types with Union Types2m 6s
-
15. Checking for Types during Runtime2m 2s
-
16. The "never" Type (added with TypeScript 2.0)1m 52s
-
17. Nullable Types (added with TypeScript 2.0)6m 22s
-
18. Module Exercise: Problem45s
-
19. Module Exercise: Solution4m 1s
Understanding the TypeScript Compiler
-
1. How Code gets Compiled1m 53s
-
2. Changing the Compiler Behavior on Errors2m 9s
-
3. Debugging your TypeScript Code using Source Maps2m 8s
-
4. Avoiding implicit "Any"1m 40s
-
5. More Compiler Options59s
-
6. Compiler Improvements with TypeScript 2.03m 7s
TypeScript and ES6
-
1. "Let" and "Const"4m 8s
-
2. Block Scope2m 41s
-
3. Arrow Functions3m 38s
-
4. Arrow Functions - Variations1m 47s
-
5. Functions and Default Parameters4m 9s
-
6. The Spread Operator3m 37s
-
7. The Rest Operator5m 13s
-
8. Destructuring Arrays3m 38s
-
9. Destructuring Objects3m 6s
-
10. Template Literals4m 5s
-
11. Other ES6 Features1m 6s
-
12. Module Exercise: Solution13m 7s
Using Classes to create Objects
-
1. Creating Classes and Class Properties8m 15s
-
2. Class Methods and Access Modifiers4m 42s
-
3. Inheritance2m 57s
-
4. Inheritance and Constructors1m 54s
-
5. Inheritance Wrap Up2m 2s
-
6. Getters & Setters5m 44s
-
7. Static Properties and Methods3m 36s
-
8. Abstract Classes5m 52s
-
9. Private Constructors & Singletons (added with TypeScript 2.0)2m 52s
-
10. "readonly" Properties (added with TypeScript 2.0)2m 12s
-
11. Module Exercise: Solution13m 21s
Namespaces and Modules
-
1. An Introduction to Namespaces6m 35s
-
2. Namespaces and Multiple Files5m 1s
-
3. Namespace Imports2m 38s
-
4. More on Namespaces2m 23s
-
5. Limitations of Namespaces1m 42s
-
6. Modules3m 58s
-
7. Loading Modules8m 57s
-
8. Importing & Exporting Modules2m 34s
-
9. Module Resolution2m 22s
-
10. Namespaces vs Modules - Wrap Up2m 7s
Doing Contract Work with Interfaces
-
1. The Basics about Interfaces5m 51s
-
2. Interfaces and Properties5m 4s
-
3. Interfaces and Methods2m 18s
-
4. Using Interfaces with Classes3m 41s
-
5. Interfaces and Function Types3m 16s
-
6. Interface Inheritance2m 18s
-
7. What happens once Interfaces get Compiled1m 39s
Generics
-
1. Why and What?4m 17s
-
2. Creating a Generic Function3m 20s
-
3. A built-in Generic Type: Arrays1m 48s
-
4. Generic Types and Arrays1m 29s
-
5. Using Generic Types2m 29s
-
6. Creating a Generic Class4m 4s
-
7. Constraints3m
-
8. Using more than one Generic type2m 42s
-
9. Module Exercise: Solution6m 11s
Behind the Scenes with Decorators
-
1. Creating a Class Decorator4m 49s
-
2. Decorator Factories3m 22s
-
3. Creating a useful Decorator3m
-
4. Using Multiple Decorators1m 2s
-
5. A first Summary2m 10s
-
6. Method Decorators5m 8s
-
7. Property Decorators4m 27s
-
8. Parameter Decorators4m 20s
Using JavaScript Libraries (like jQuery) with TypeScript
-
1. Installing a Third-Party Library1m 12s
-
2. Importing the Library4m 42s
-
3. Translating JavaScript to TypeScript with TypeScript Definition Files2m 6s
-
4. Option 1: Manually download TypeScript Definition Files2m 18s
-
5. Option 2: Managing TypeScript Defintion Files with the "typings" Package3m 28s
-
6. Easier Type Management with TypeScript 2.03m 8s
TypeScript Workflows
-
1. Using "tsc" and the tsconfig File2m 54s
-
2. How TypeScript resolves Files using the tsconfig.json File5m 52s
-
3. More on "tsc" and the tsconfig File2m 57s
-
4. Adding TypeScript into a Gulp Workflow8m 2s
-
5. Adding TypeScript into a Webpack Workflow10m 43s
Example: Using TypeScript together with ReactJS
-
1. Setting up the Project & Adding React Packages2m 56s
-
2. Adding the ReactJS TypeScript Definition Files2m 1s
-
3. Installing Webpack1m 46s
-
4. Configuring Webpack3m 50s
-
5. Creating ReactJS Code - with TypeScript6m 15s
-
6. Configuring the TypeScript Compiler to work with ReactJS2m 57s
-
7. Using TypeScript 2.0 Type Management2m 31s