Spring MVC and Hibernate CRUD Complete Guide
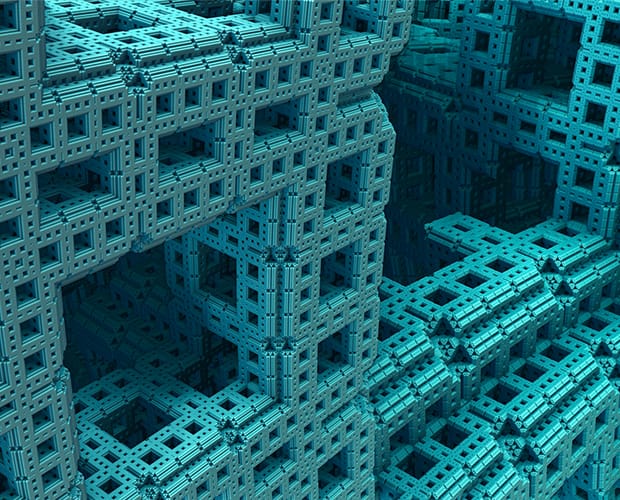
Get ready for your exam by enrolling in our comprehensive training course. This course includes a full set of instructional videos designed to equip you with in-depth knowledge essential for passing the certification exam with flying colors.
$14.99 / $24.99
Course Introduction
-
1. Introduction2m
-
2. Practice Activities - Overview1m
Spring Overview
-
1. Why Spring? - part 14m
-
2. Why Spring? - part 25m
-
3. Spring Core Framework - Part 14m
-
4. Spring Core Framework - Part 23m
-
5. Spring Platform3m
Setting Up Your Development Environment
-
1. Dev Environment Overview2m
-
2. Installing Tomcat5m
-
3. Installing Eclipse5m
-
4. Connecting Tomcat to Eclipse3m
-
5. Downloading Spring 5 JAR Files - Overview1m
-
6. Downloading Spring 5 JAR files - Installation6m
Spring Inversion of Control - XML Configuration
-
1. What is Inversion of Control?3m
-
2. Code Demo - Rough Prototype Part 17m
-
3. Code Demo - Rough Prototype Part 24m
-
4. Spring Inversion of Control - Overview6m
-
5. Spring Inversion of Control - Write Some Code - Part 18m
-
6. Spring Inversion of Control - Write Some Code - Part 22m
Spring Dependency Injection - XML Configuration
-
1. Spring Dependency Injection - Overview8m
-
2. Spring Dependency Injection - Behind the Scenes2m
-
3. Spring Dependency Injection - Write Some Code - Part 14m
-
4. Spring Dependency Injection - Write Some Code - Part 26m
-
5. Spring Dependency Injection - Write Some Code - Part 37m
-
6. Setter Injection - Overview3m
-
7. Setter Injection - Write Some Code - Part 15m
-
8. Setter Injection - Write Some Code - Part 28m
-
9. Injecting Literal Values - Overview2m
-
10. Injecting Literal Values - Write Some Code7m
-
11. Injecting Values from a Properties File - Overview3m
-
12. Injecting Values from a Properties File - Write Some Code6m
Spring Bean Scopes and Lifecycle
-
1. Bean Scopes - Overview4m
-
2. Bean Scopes - Write Some Code - Part 16m
-
3. Bean Scopes - Write Some Code - Part 25m
-
4. Bean Lifecycle - Overview3m
-
5. Bean Lifecycle - Write Some Code8m
Spring Configuration with Java Annotations - Inversion of Control
-
1. Annotations Overview - Component Scanning7m
-
2. Annotations Project Setup3m
-
3. Explicit Component Names - Write Some Code - Part 14m
-
4. Explicit Component Names - Write Some Code - Part 28m
-
5. Default Component Names - Overview2m
-
6. Default Component Names - Write Some Code5m
Spring Configuration with Java Annotations - Dependency Injection
-
1. Constructor Injection - Overview6m
-
2. Constructor Injection - Write Some Code - Part 13m
-
3. Constructor Injection - Write Some Code - Part 25m
-
4. Setter Injection - Overview2m
-
5. Setter Injection - Write Some Code5m
-
6. Method Injection3m
-
7. Field Injection - Overview2m
-
8. Field Injection - Write Some Code3m
-
9. Which Injection Type Should You Use?2m
-
10. Qualifiers for Dependency Injection - Overview3m
-
11. Qualifiers for Dependency Injection - Write Some Code - Part 17m
-
12. Qualifiers for Dependency Injection - Write Some Code - Part 26m
Spring Configuration with Java Annotations - Bean Scopes and Lifecycle Methods
-
1. @Scope Annotation - Overview2m
-
2. @Scope Annotation - Write Some Code7m
-
3. Bean Lifecycle Method Annotations - Overview1m
-
4. Bean Lifecycle Method Annotations - Write Some Code4m
Spring Configuration with Java Code (no xml)
-
1. Spring Configuration with Java Code (no xml) - Overview4m
-
2. Spring Configuration with Java Code (no xml) - Write Some Code6m
-
3. Defining Spring Beans with Java Code (no xml) - Overview4m
-
4. Defining Spring Beans with Java Code (no xml) - Write Some Code - Part 14m
-
5. Defining Spring Beans with Java Code (no xml) - Write Some Code - Part 25m
-
6. Injecting Values from Properties File - Overview3m
-
7. Injecting Values from Properties File - Write Some Code - Part 12m
-
8. Injecting Values from Properties File - Write Some Code - Part 25m
Spring MVC - Building Spring Web Apps
-
1. Spring MVC Overview4m
-
2. Spring MVC - Behind the Scenes5m
-
3. Development Environment Checkpoint1m
-
4. Spring MVC Configuration - Overview5m
-
5. Spring MVC Configuration - JAR Files4m
-
6. Spring MVC Configuration - Config Files4m
Spring MVC - Creating Controllers and Views
-
1. Creating a Spring Home Controller and View - Overview5m
-
2. Creating a Spring Home Controller and View - Write Some Code - Part 15m
-
3. Creating a Spring Home Controller and View - Write Some Code - Part 22m
-
4. Reading HTML Form Data - Overview3m
-
5. Reading HTML Form Data - Write Some Code - Part 15m
-
6. Reading HTML Form Data - Write Some Code - Part 24m
-
7. Reading HTML Form Data - Write Some Code - Part 33m
-
8. Adding Data to the Spring Model - Overview6m
-
9. Adding Data to the Spring Model - Write Some Code - Part 14m
-
10. Adding Data to the Spring Model - Write Some Code - Part 24m
Spring MVC - Request Params and Request Mappings
-
1. Binding Request Params - Overview2m
-
2. Binding Request Params - Write Some Code5m
-
3. Controller Level Request Mapping - Overview2m
-
4. Controller Level Request Mapping - Write Some Code - Part 14m
-
5. Controller Level Request Mapping - Write Some Code - Part 24m
Spring MVC - Form Tags and Data Binding
-
1. Spring MVC Form Tags Overview3m
-
2. Text Fields - Overview6m
-
3. Text Fields - Write Some Code - Part 16m
-
4. Text Fields - Write Some Code - Part 26m
-
5. Text Fields - Write Some Code - Part 34m
-
6. Drop-Down Lists - Overview2m
-
7. Drop-Down Lists - Write Some Code - Part 15m
-
8. Drop-Down Lists - Write Some Code - Part 25m
-
9. Radio Buttons - Overview2m
-
10. Radio Buttons - Write Some Code5m
-
11. Checkboxes - Overview1m
-
12. Checkboxes - Write Some Code - Part 15m
-
13. Checkboxes - Write Some Code - Part 23m
Spring MVC Form Validation - Applying Built-In Validation Rules
-
1. Spring MVC Form Validation Overview3m
-
2. Setting Up Dev Environment for Form Validation2m
-
3. Installing Validation Files3m
-
4. Checking for Required Fields Overview5m
-
5. Add Validation Rule to Customer Class3m
-
6. Display Validation Error Messages on HTML Form7m
-
7. Perform Validation in Controller Class - Part 14m
-
8. Perform Validation in Controller Class - Part 24m
-
9. Update Confirmation Page4m
-
10. Test the Validation Rule for Required Fields3m
-
11. Add Pre-processing Code with @InitBinder - Overview4m
-
12. Add Pre-processing Code with @InitBinder - Write Some Code5m
Spring MVC Form Validation - Validating Number Ranges and Regular Expressions
-
1. Validating a Number Range - Overview3m
-
2. Validating a Number Range - Write Some Code7m
-
3. Applying Regular Expressions - Overview2m
-
4. Applying Regular Expressions - Write Some Code6m
-
5. How to make Integer Field Required: freePasses5m
-
6. How to Handle String input for Integer Fields - Custom Message4m
-
7. How to Handle String input for Integer Fields - Configure Resource Bundle4m
-
8. How to Handle String input for Integer Fields - Deep Dive5m
Spring MVC Form Validation - Creating Custom Validation Rules
-
1. Custom Form Validation - Overview - Part 14m
-
2. Custom Form Validation - Overview - Part 26m
-
3. Creating a Custom Java Annotation - Part 14m
-
4. Creating a Custom Java Annotation - Part 23m
-
5. Developing the ConstraintValidator5m
-
6. Adding Validation Rule to the Entity and Form4m
-
7. Testing the Custom Validation Rule5m
Introduction to Hibernate
-
1. Hibernate Overview8m
-
2. Hibernate and JDBC1m
Setting Up Hibernate Development Environment
-
1. Hibernate Development Environment Overview1m
-
2. Installing MySQL on MS Windows5m
-
3. Setting Up Database Table7m
-
4. Setting up Hibernate in Eclipse9m
-
5. Testing Your JDBC Connection6m
Hibernate Configuration with Annotations
-
1. Hibernate Development Process Overview1m
-
2. Creating the Hibernate Configuration File5m
-
3. Hibernate Annotations - Part 17m
-
4. Hibernate Annotations - Part 27m
Hibernate CRUD Features: Create, Read, Update and Delete
-
1. Creating and Saving Java Objects - Part 16m
-
2. Creating and Saving Java Objects - Part 29m
-
3. Primary Keys - Overview6m
-
4. Primary Keys - Write Some Code7m
-
5. Primary Keys - Changing the Starting Index3m
-
6. Reading Objects with Hibernate10m
-
7. Querying Objects with Hibernate - Overview4m
-
8. Querying Objects with Hibernate - Write Some Code - Part 17m
-
9. Querying Objects with Hibernate - Write Some Code - Part 27m
-
10. Updating Objects with Hibernate - Overview4m
-
11. Updating Objects with Hibernate - Write Some Code8m
-
12. Deleting Objects with Hibernate - Overview3m
-
13. Deleting Objects with Hibernate - Write Some Code7m
Hibernate Advanced Mappings
-
1. Advanced Mappings Overview3m
-
2. Database Concepts5m
Hibernate Advanced Mappings - @OneToOne
-
1. @OneToOne - Overview - Part 15m
-
2. @OneToOne - Overview - Part 26m
-
3. @OneToOne - Overview - Part 34m
-
4. @OneToOne - Run Database Scripts7m
-
5. @OneToOne - Write Some Code - Prep Work4m
-
6. @OneToOne - Write Some Code - Create InstructorDetail class6m
-
7. @OneToOne - Write Some Code - Create Instructor class7m
-
8. @OneToOne - Write Some Code - Build Main App - Part 14m
-
9. @OneToOne - Write Some Code - Build Main App - Part 25m
-
10. @OneToOne - Delete an Entity5m
Hibernate Advanced Mappings - @OneToMany
-
1. @OneToMany - Bi-Directional Overview - Part 14m
-
2. @OneToMany - Bi-Directional Overview - Part 24m
-
3. @OneToMany - Bi-Directional - Database Prep Work5m
-
4. @OneToMany - Bi-Directional - Create Course Mapping5m
-
5. @OneToMany - Bi-Directional - Define Course Relationship3m
-
6. @OneToMany - Bi-Directional - Update Instructor5m
-
7. @OneToMany - Bi-Directional - Add Instructor to Database5m
-
8. @OneToMany - Bi-Directional - Create Courses for Instructor5m
-
9. @OneToMany - Bi-Directional - Retrieve Instructor Courses4m
-
10. @OneToMany - Bi-Directional - Delete a Course4m
Hibernate Advanced Mappings - Eager vs Lazy Loading
-
1. Eager vs Lazy Loading - Overview - Part 13m
-
2. Eager vs Lazy Loading - Overview - Part 25m
-
3. Eager vs Lazy Loading - Coding - Eager8m
-
4. Eager vs Lazy Loading - Coding - Lazy4m
-
5. Eager vs Lazy Loading - Coding - Closing the Session4m
-
6. Eager vs Lazy Loading - Coding - Resolve Lazy Loading Issue3m
-
7. Eager vs Lazy Loading - Coding - HQL JOIN FETCH7m
Hibernate Advanced Mappings - @OneToMany - Unidirectional
-
1. @OneToMany - Uni-Directional - Overview - Part 14m
-
2. @OneToMany - Uni-Directional - Overview - Part 23m
-
3. @OneToMany - Uni-Directional - Set up database tables4m
-
4. @OneToMany - Uni-Directional - Create Review Class6m
-
5. @OneToMany - Uni-Directional - Configure Fetch Type5m
-
6. @OneToMany - Uni-Directional - Create Course Reviews8m
-
7. @OneToMany - Uni-Directional - Get Course Reviews4m
-
8. @OneToMany - Uni-Directional - Delete Course Reviews3m
Hibernate Advanced Mappings - @ManyToMany
-
1. @ManyToMany - Overview - Part 15m
-
2. @ManyToMany - Overview - Part 26m
-
3. @ManyToMany - Set up database tables4m
-
4. @ManyToMany - Update Course class4m
-
5. @ManyToMany - Configure Course for many-to-many5m
-
6. @ManyToMany - Configure Student for many-to-many3m
-
7. @ManyToMany - Create a Main App5m
-
8. @ManyToMany - Review app output2m
-
9. @ManyToMany - Add more courses for a student4m
-
10. @ManyToMany - Verify Data in Join Table4m
-
11. @ManyToMany - Get Courses for Student4m
-
12. @ManyToMany - Delete a Course6m
-
13. @ManyToMany - Delete a Student4m
Build a Database Web App - Spring MVC and Hibernate Project - Part 1
-
1. Project Overview and Demo4m
-
2. Set up Sample Data for Database6m
-
3. Test Database Connection - Part 14m
-
4. Test Database Connection - Part 25m
-
5. Set Up Dev Environment - Part 15m
-
6. Set Up Dev Environment - Part 24m
-
7. Set Up Dev Environment - Part 32m
-
8. Test Spring MVC Controller - Part 12m
-
9. Test Spring MVC Controller - Part 26m
Build a Database Web App - Spring MVC and Hibernate Project - Part 2
-
1. List Customers - Overview3m
-
2. List Customers - Overview of Development Process1m
-
3. List Customers - Creating Hibernate Entity - Part 13m
-
4. List Customers - Creating Hibernate Entity - Part 26m
-
5. List Customers - Developing Hibernate DAO - Overview - Part 16m
-
6. List Customers - Developing Hibernate DAO - Overview - Part 23m
-
7. List Customers - Developing Hibernate DAO - Write Some Code - Part 12m
-
8. List Customers - Developing Hibernate DAO - Write Some Code - Part 27m
-
9. List Customers - Injecting DAO into Controller4m
-
10. List Customers - Developing JSP View Page6m
-
11. Making it Pretty with CSS - Overview5m
-
12. Making it Pretty with CSS - Write Some Code - Part 13m
-
13. Making it Pretty with CSS - Write Some Code - Part 25m
-
14. Adding a Welcome File3m
Build a Database Web App - Spring MVC and Hibernate Project - Part 3
-
1. Refactor: @GetMapping and @PostMapping - Overview5m
-
2. Refactor: @GetMapping and @PostMapping - Write Some Code3m
-
3. Refactor: Add a Service Layer - Overview5m
-
4. Refactor: Add a Service Layer - Write Some Code - Part 15m
-
5. Refactor: Add a Service Layer - Write Some Code - Part 23m
Build a Database Web App - Spring MVC and Hibernate Project - Part 4
-
1. Add Customer - Overview2m
-
2. Add Customer - Setting up the Add Button - Part 14m
-
3. Add Customer - Setting up the Add Button - Part 23m
-
4. Add Customer - Creating the HTML Form - Part 11m
-
5. Add Customer - Creating the HTML Form - Part 25m
-
6. Add Customer - Creating the HTML Form - Part 34m
-
7. Add Customer - Save to Database - Part 15m
-
8. Add Customer - Save to Database - Part 24m
-
9. Sort Customer Data2m
Build a Database Web App - Spring MVC and Hibernate Project - Part 5
-
1. Update Customer - Overview2m
-
2. Update Customer - Creating Update Link5m
-
3. Update Customer - Prepopulating the Form - Part 13m
-
4. Update Customer - Prepopulating the Form - Part 24m
-
5. Update Customer - Prepopulating the Form - Part 33m
-
6. Update Customer - Save Customer to Database - Part 14m
-
7. Update Customer - Save Customer to Database - Part 22m
Build a Database Web App - Spring MVC and Hibernate Project - Part 6
-
1. Delete Customer - Overview2m
-
2. Delete Customer - Creating Delete Link - Part 14m
-
3. Delete Customer - Creating Delete Link - Part 23m
-
4. Delete Customer - Delete from Database - Part 14m
-
5. Delete Customer - Delete from Database - Part 23m
-
6. Delete Customer - Delete from Database - Part 33m
AOP: Aspect-Oriented Programming Overview
-
1. AOP - The Business Problem9m
-
2. AOP Solution and AOP Use Cases5m
-
3. Comparing Spring AOP and AspectJ7m
AOP: @Before Advice Type
-
1. AOP: @Before Advice Overview4m
-
2. AOP: @Before Advice Overview 26m
-
3. AOP: AOP Project Setup6m
-
4. AOP: @Before Advice - Write Some Code5m
-
5. AOP: @Before Advice - Write Some Code 25m
-
6. AOP: @Before Advice - Add and Test AOP Aspect5m
-
7. AOP: @Before Advice - Add and Test AOP Aspect 25m
AOP: Pointcut Expressions - Match Methods and Return Types
-
1. AOP - Pointcut Expressions Overview4m
-
2. AOP - Pointcut Expressions Overview 23m
-
3. AOP - Pointcut Expressions - Match any addAccount Method - Part 24m
-
5. AOP - Pointcut Expressions - Match only DAO addAccount - Match any add* Method4m
-
6. AOP - Pointcut Expressions - Match only DAO addAccount - Match any add* Method 23m
-
7. AOP - Pointcut Expressions - Match any Return Type4m
AOP: Pointcut Expressions - Match Method Parameter Types
-
1. AOP: Pointcut Expressions - Match Method Parameter Types Overview4m
-
2. AOP: Pointcut Expressions - Match Method with Account and more Params7m
-
3. AOP: Pointcut Expressions - Match Method with Account and more Params 23m
-
4. AOP: Pointcut Expressions - Match Method Any Params - Match Method in a Package2m
-
5. AOP: Pointcut Expressions - Match Method Any Params - Match Method in a Package 26m
AOP: Pointcut Declarations
-
1. AOP: Pointcut Declarations - Overview4m
-
2. AOP: Pointcut Declarations - Write Some Code6m
-
3. AOP: Pointcut Declarations - Write Some Code 23m
-
4. AOP: Combining Pointcuts - Overview5m
-
5. AOP: Combining Pointcuts - Write Some Code - Part 17m
-
6. AOP: Combining Pointcuts - Write Some Code - Part 27m
AOP: Ordering Aspects
-
1. AOP: Ordering Aspects - Overview7m
-
2. AOP: Ordering Aspects - Write Some Code - Part 16m
-
3. AOP: Ordering Aspects - Write Some Code - Part 1/25m
-
4. AOP: Ordering Aspects - Write Some Code - Part 26m
AOP: JoinPoints
-
1. AOP: Read Method Arguments with JoinPoints - Overview3m
-
2. AOP: Read Method Arguments with JoinPoints - Overview 26m
-
3. AOP: Read Method Arguments with JoinPoints - Write Some Code6m
AOP: @AfterReturning Advice Type
-
1. AOP: @AfterReturning Overview1m
-
2. AOP: @AfterReturning Overview 26m
-
3. AOP: @AfterReturning - Write Some Code - Part 14m
-
4. AOP: @AfterReturning - Write Some Code - Part 1/24m
-
5. AOP: @AfterReturning - Write Some Code - Part 25m
-
6. AOP: @AfterReturning - Write Some Code - Part 37m
-
7. AOP: @AfterReturning - Modifying Data - Write Some Code4m
-
8. AOP: @AfterReturning - Modifying Data - Write Some Code 26m
AOP: @AfterThrowing Advice Type
-
1. AOP: @AfterThrowing7m
-
2. AOP: @AfterThrowing 24m
-
3. AOP: @AfterThrowing - Write Some Code5m
-
4. AOP: @AfterThrowing - Write Some Code 26m
AOP: @After Advice Type
-
1. AOP: @After Overview4m
-
2. AOP: @After - Write Some Code6m
-
3. AOP: @After - Write Some Code 23m
@Around Advice Type
-
1. AOP: @Around Advice Overview6m
-
2. AOP: @Around - Write Some Code - Part 14m
-
3. AOP: @Around - Write Some Code - Part 1/25m
-
4. AOP: @Around - Write Some Code - Part 23m
-
5. AOP: @Around - Write Some Code - Part 2/24m
-
6. AOP: @Around Advice - Resolve Order Issue5m
-
7. AOP: @Around Advice - Resolve Order Issue 24m
-
8. AOP: @Around Advice - Handling Exceptions - Overview5m
-
9. AOP: @Around Advice - Handling Exceptions - Write Some Code6m
-
10. AOP: @Around Advice - Handling Exceptions - Write Some Code 25m
-
11. AOP: @Around Advice - Rethrowing Exceptions5m
AOP: Add AOP Logging to Spring MVC App - Real-Time Project
-
1. AOP: AOP and Spring MVC App - Overview5m
-
2. AOP: AOP and Spring MVC App - Write Some Code - Create Aspect6m
-
3. AOP: AOP and Spring MVC App - Write Some Code - Create Aspect 23m
-
4. AOP: AOP and Spring MVC App - Write Some Code - Add @Before Advice6m
-
5. AOP: AOP and Spring MVC App - Write Some Code - Add @Before Advice 23m
-
6. AOP: AOP and Spring MVC App - Write Some Code - Add @AfterReturning Advice5m