Build a Real-World Project Using JavaScript
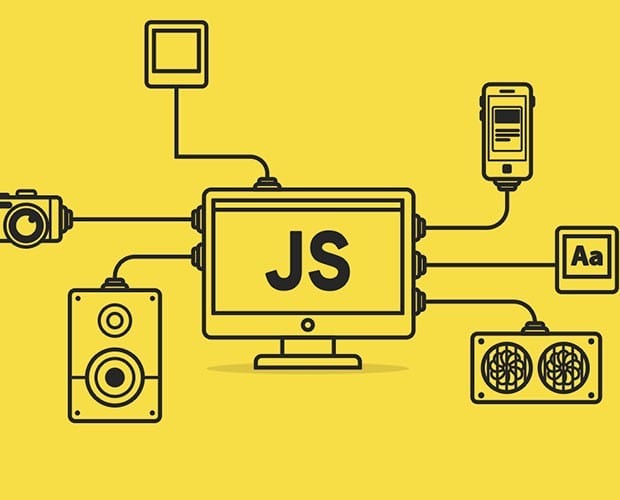
Get ready for your exam by enrolling in our comprehensive training course. This course includes a full set of instructional videos designed to equip you with in-depth knowledge essential for passing the certification exam with flying colors.
$14.99 / $24.99
Course Introduction
-
1. Setting up Our Tools: Brackets and Google Chrome3m 48s
JavaScript Language Basics
-
1. Section Intro49s
-
2. Introduction to JavaScript4m 7s
-
3. Getting Started with JavaScript4m 24s
-
4. Variables and Data Types8m 43s
-
5. Variable Mutation and Type Coercion15m 13s
-
6. Operators13m 57s
-
7. If / else Statements8m 56s
-
8. Boolean Logic and Switch Statements15m 48s
-
9. Coding Challenge 11m 39s
-
10. Coding Challenge 1: Solution13m 51s
-
11. Functions15m 31s
-
12. Statements and Expressions2m 44s
-
13. Arrays11m 10s
-
14. Objects and Properties9m
-
15. Objects and Methods14m 55s
-
16. Loops and Iteration19m 6s
-
17. Coding Challenge 22m 52s
-
18. Coding Challenge 2: Solution13m 7s
-
19. Important Note: ES5, ES6 / ES2015 and ES20164m 47s
How JavaScript Works Behind the Scenes
-
1. Section Intro1m 7s
-
2. How Our Code Is Executed: JavaScript Parsers and Engines2m 33s
-
3. Execution Contexts and the Execution Stack5m 38s
-
4. Execution Contexts in Detail: Creation and Execution Phases and Hoisting4m 5s
-
5. Hoisting in Practice12m 6s
-
6. Scoping and the Scope Chain12m 44s
-
7. The 'this' Keyword1m 38s
-
8. The 'this' Keyword in Practice11m 11s
JavaScript in the Browser: DOM Manipulation and Events
-
1. Section Intro40s
-
2. The DOM and DOM Manipulation1m 47s
-
3. 5-Minute HTML and CSS Crash Course5m 6s
-
4. Project Setup and Details4m 8s
-
5. First DOM Access and Manipulation19m 33s
-
6. Events and Event Handling: Rolling the Dice17m 17s
-
7. Updating Scores and Changing the Active Player13m 54s
-
8. Implementing Our 'Hold' Function and the DRY Principle17m 11s
-
9. Creating a Game Initialization Function11m 10s
-
10. Finishing Touches: State Variables7m 30s
-
11. Coding Challenge 32m 12s
-
12. Coding Challenge 3: Solution, Part 16m 3s
-
13. Coding Challenge 3: Solution, Part 28m 51s
-
14. Coding Challenge 3: Solution, Part 36m 56s
Advanced JavaScript: Objects and Functions
-
1. Section Intro1m 2s
-
2. Everything Is an Object: Inheritance and the Prototype Chain9m 45s
-
3. Creating Objects: Function Constructors14m 6s
-
4. The Prototype Chain in the Console8m 19s
-
5. Creating Objects: Object.create6m 26s
-
6. Primitives vs8m 11s
-
7. First Class Functions: Passing Functions as Arguments14m 44s
-
8. First Class Functions: Functions Returning Functions8m 27s
-
9. Immediately Invoked Function Expressions (IIFE)6m 19s
-
10. Closures16m 24s
-
11. Bind, Call and Apply16m 44s
-
12. Coding Challenge 46m 49s
-
13. Coding Challenge 4: Solution, Part 117m 47s
-
14. Coding Challenge 4: Solution, Part 215m 46s
Putting It All Together: The Budget App Project
-
1. Section Intro1m 11s
-
2. Project Setup and Details4m 26s
-
3. Project Planning and Architecture: Step 15m 17s
-
4. Implementing the Module Pattern17m 12s
-
5. Setting up the First Event Listeners15m 43s
-
6. Reading Input Data16m 10s
-
7. Creating an Initialization Function5m 9s
-
8. Creating Income and Expense Function Constructors9m 21s
-
9. Adding a New Item to Our Budget Controller18m 2s
-
10. Adding a New Item to the UI19m 28s
-
11. Clearing Our Input Fields11m 23s
-
12. Updating the Budget: Controller10m 54s
-
13. Updating the Budget: Budget Controller20m 38s
-
14. Updating the Budget: UI Controller11m 41s
-
15. Project Planning and Architecture: Step 22m 16s
-
16. Event Delegation3m 36s
-
17. Setting up the Delete Event Listener Using Event Delegation19m 13s
-
18. Deleting an Item from Our Budget Controller17m 9s
-
19. Deleting an Item from the UI7m 51s
-
20. Project Planning and Architecture: Step 31m 46s
-
21. Updating the Percentages: Controller3m 37s
-
22. Updating the Percentages: Budget Controller14m 21s
-
23. Updating the Percentages: UI Controller11m 49s
-
24. Formatting Our Budget Numbers: String Manipulation19m
-
25. Displaying the Current Month and Year6m 48s
-
26. Finishing Touches: Improving the UX10m 17s
-
27. We’ve Made It! Final Considerations1m 10s
Get Ready for the Future: ES6 / ES2015
-
1. What's new in ES6 / ES20154m 43s
-
2. Variable Declarations with let and const16m 17s
-
3. Blocks and IIFEs3m 38s
-
4. Strings in ES6 / ES201510m 2s
-
5. Arrow Functions: Basics8m 4s
-
6. Arrow Functions: Lexical 'this' Keyword19m 11s
-
7. Destructuring7m 51s
-
8. Arrays in ES6 / ES201517m 19s
-
9. The Spread Operator9m 56s
-
10. Rest Parameters13m 23s
-
11. Default Parameters7m 48s
-
12. Maps19m 53s
-
13. Classes9m 47s
-
14. Classes with Subclasses15m 47s
-
15. Coding Challenge 52m 46s
-
16. Coding Challenge 5: Solution30m 58s
-
17. How to use ES2015 / ES6 Today!14m 9s
Conclusion
-
1. Where to Go from Here1m 45s