Fundamentals of React and Redux
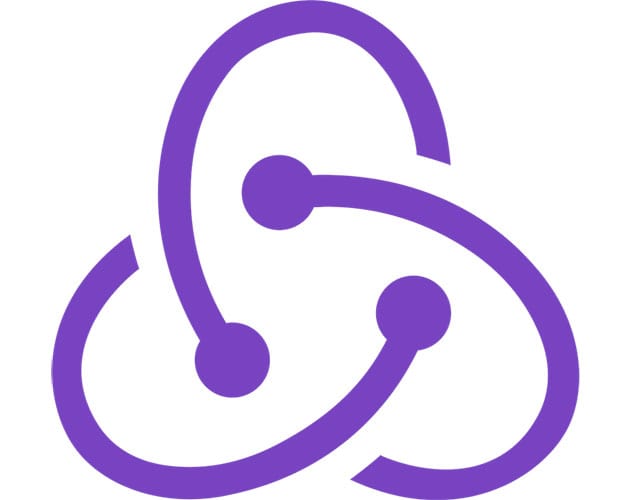
Get ready for your exam by enrolling in our comprehensive training course. This course includes a full set of instructional videos designed to equip you with in-depth knowledge essential for passing the certification exam with flying colors.
$14.99 / $24.99
An Intro to React
-
1. Environment Setup3m 42s
-
2. Project Setup1m 45s
-
3. A Taste of JSX2m 53s
-
4. More on JSX11m 29s
-
5. ES6 Import Statements4m 35s
-
6. ReactDOM vs React3m 59s
-
7. Differences Between Component Instances and Component Classes2m 26s
-
8. Render Targets3m 38s
-
9. Component Structure5m 53s
-
10. Youtube Search API Signup6m 26s
-
11. Export Statements6m 39s
-
12. Class-Based Components8m 40s
-
13. Handling User Events8m 11s
-
14. Introduction to State9m 30s
-
15. More on State5m 13s
-
16. Controlled Components6m 4s
-
17. Breather and Review7m 47s
Ajax Requests with React
-
1. Youtube Search Response4m 26s
-
2. Refactoring Functional Components to Class Components7m 43s
-
3. Props7m 42s
-
4. Building Lists with Map7m 6s
-
5. List Item Keys3m 54s
-
6. Video List Items7m 53s
-
7. Detail Component and Template Strings7m 5s
-
8. Handling Null Props4m 41s
-
9. Video Selection11m 39s
-
10. Styling with CSS3m 43s
-
11. Searching for Videos7m 51s
-
12. Throttling Search Term Input5m 46s
-
13. React Wrapup5m 24s
Modeling Application State
-
1. Foreword on Redux2m 16s
-
2. What is Redux?2m 45s
-
3. More on Redux3m 42s
-
4. Even More on Redux!4m 19s
Managing App State with Redux
-
1. Reducers7m 43s
-
2. Containers - Connecting Redux to React7m 23s
-
3. Containers Continued4m
-
4. Implementation of a Container Class10m 46s
-
5. Containers and Reducers Review4m 9s
-
6. Actions and Action Creators7m 31s
-
7. Binding Action Creators9m 41s
-
8. Creating an Action6m 45s
-
9. Consuming Actions in Reducers7m 16s
-
10. Consuming Actions in Reducers Continued5m 5s
-
11. Conditional Rendering6m 38s
-
12. Reducers and Actions Review5m 2s
Intermediate Redux: Middleware
-
1. App Overview and Planning5m 10s
-
2. Component Setup7m 57s
-
3. Controlled Components and Binding Context9m 56s
-
4. Form Elements in React6m 29s
-
5. Working with API's8m 12s
-
6. Introduction to Middleware7m 27s
-
7. Ajax Requests with Axios13m 3s
-
8. Redux-Promise in Practice10m 15s
-
9. Redux-Promise Continued10m 55s
-
10. Avoiding State Mutations in Reducers9m 53s
-
11. Building a List Container6m 54s
-
12. Mapping Props to a Render Helper6m 7s
-
13. Adding Sparkline Charts9m 55s
-
14. Making a Reusable Chart Component6m
-
15. Labeling of Units10m 37s
-
16. Google Maps Integration7m 59s
-
17. Google Maps Integration Continued7m 16s
-
18. Project Review4m 59s
React Router + Redux Form
-
1. App Overview and Goals6m 49s
-
2. Exploring the Posts Api13m 26s
-
3. Installing React Router2m 36s
-
4. React Router - What is It?5m 34s
-
5. Setting Up React Router4m 39s
-
6. Route Configuration5m 16s
-
7. Nesting Of Routes8m 2s
-
8. IndexRoutes with React Router5m 4s
-
9. Back To Redux - Index Action5m 50s
-
10. Catching Data with Posts Reducer6m 29s
-
11. Catching Data with Posts Reducer Continued2m 38s
-
12. Fetching Data with Lifecycle Methods5m 43s
-
13. Fetching Data with Lifecycle Methods Continued6m 50s
-
14. Creating New Posts5m 23s
-
15. Navigation with the Link Component4m 26s
-
16. Forms and Form Submission10m 12s
-
17. More on Forms and Form Submission7m 37s
-
18. Passing Control to Redux Form7m 51s
-
19. CreatePost Action Creator11m 21s
-
20. Form Validation8m 28s
-
21. Form Validation Continued8m 51s
-
22. Navigating on Submit12m 37s
-
23. Posts Index4m 31s
-
24. Dynamic Route Params7m 9s
-
25. Loading Data on Render5m 45s
-
26. Handling Null Props5m 31s
-
27. Delete Action Creator7m 7s
-
28. Navigate on Delete2m 25s
-
29. ReactRouter and ReduxForm Wrapup7m 2s
-
2. Posts API9m 20s
-
4. What React Router Does5m 36s
-
5. The Basics of React Router8m 59s
-
6. Route Design6m 31s
-
7. Our First Route Definition5m 57s
-
8. State as an Object9m 7s
-
10. Implementing Posts Reducer10m 29s
-
11. Action Creator Shortcuts8m 6s
-
12. Rendering a List of Posts9m 19s
-
14. A React Router Gotcha4m 45s
-
16. Redux Form5m 33s
-
17. Setting Up Redux Form9m 27s
-
18. The Field Component10m 49s
-
19. Generalizing Fields8m 54s
-
20. Validating Forms10m 31s
-
21. Showing Errors to Users4m 30s
-
22. Handling Form Submittal9m 31s
-
23. Form and Field States6m 7s
-
24. Conditional Styling7m 6s
-
25. More on Navigation3m 12s
-
26. Create Post Action Creator10m 5s
-
27. Navigation Through Callbacks7m 31s
-
28. The Posts Show Component3m 39s
-
29. Receiving New Posts9m 26s
-
30. Selecting from OwnProps11m 27s
-
31. Data Dependencies5m 32s
-
32. Caching Records6m 13s
-
33. Deleting a Post9m 25s
-
34. Wrapup9m 10s
Bonus - RallyCoding
-
1. Basics of Redux Thunk7m 17s
-
2. Combining Redux and Firebase12m 17s
-
3. Dynamic Forms with Redux Form14m 42s
-
4. Logicless Components with Reselect18m 9s
-
5. Data Loading Methods with Redux9m 29s
-
6. Animation of React Components12m 33s
-
7. The Best Way to Store Redux Data12m 2s
-
8. Four Most Common Errors with React and Redux18m 43s
-
9. Modals in React and Redux Apps15m 5s
-
10. React Integration with 3rd Party Libraries12m 18s
-
11. Introducing JSPlaygrounds for Rapid Prototyping23m 15s