Python And PostgresSQL: Complete Guide
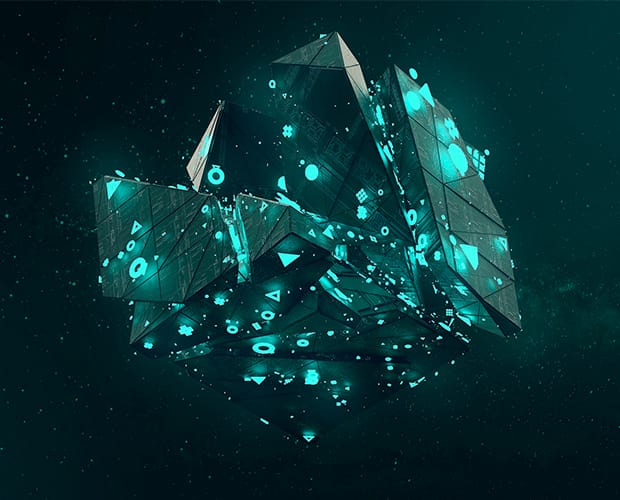
Get ready for your exam by enrolling in our comprehensive training course. This course includes a full set of instructional videos designed to equip you with in-depth knowledge essential for passing the certification exam with flying colors.
$14.99 / $24.99
Installing Python
-
1. Installing Python on Mac1m
Python 101
-
1. The Python interactive shell (IDLE)4m
-
2. Numbers and Strings in Python5m
-
3. Variables in Python6m
-
4. The str() method to convert to Strings4m
-
5. Running a Python file from the IDLE7m
-
6. Getting user input in Python6m
-
7. The int() method to convert to Integers2m
-
8. The format() method to simplify String formatting7m
Magic Numbers in Python
-
1. Lists in Python5m
-
2. The for loop in Python3m
-
3. Boolean expressions, True and False5m
-
4. If statements in Python5m
-
5. The 'in' keyword to check whether a list contains an element5m
-
6. Giving the user multiple chances in our program9m
-
7. Generating random integers in Python8m
-
8. Defining our own methods in Python7m
-
9. Returning values from our methods in Python5m
A Lottery app
-
1. The String split() method4m
-
2. List comprehension in Python7m
-
3. Python Sets — what is a Set?7m
-
4. Set comprehension in Python6m
-
5. Creating our lottery numbers8m
-
6. Calculating the lottery winnings6m
More complex applications: a student registration system
-
1. Installing PyCharm, a professional Python tool3m
-
2. Setting up PyCharm on Mac2m
-
3. Setting up PyCharm on Windows4m
-
4. Dictionaries in Python9m
-
5. Advanced Dictionary usage in Python8m
-
6. Methods returning dictionaries7m
-
7. Appending to a list in Python5m
-
8. Adding marks to our student data structure9m
-
9. The sum() method in Python8m
-
10. Iterating over a list and using the dictionaries inside it6m
-
11. Creating the application menu15m
Object-Oriented Programming: a movie rental system
-
1. What are classes? Classes in Python12m
-
2. The Movie Class8m
-
3. The User Class (and the __repr__ method)6m
-
4. The filter() method in Python10m
-
5. More Movie operations and methods6m
-
6. Writing to a file in Python4m
-
7. Saving CSV files with our data10m
-
8. Loading our data from CSV files12m
-
9. Saving JSON files with our data6m
-
10. Loading our data from JSON files8m
-
11. Argument unpacking in Python (the two asterisks)6m
-
12. Creating the menu for our application15m
Introduction to PostgreSQL
-
1. Introduction to databases13m
-
2. Installing PostgreSQL on Windows4m
-
3. Using PostgreSQL on Windows7m
-
4. Executing SQL queries on Windows2m
-
5. Installing PostgreSQL on Mac5m
-
6. Using PostgreSQL on Mac9m
-
7. Executing SQL commands on Mac1m
-
8. Using the sample data provided3m
-
9. SQL: The SELECT command8m
-
10. SQL: filtering with WHERE4m
-
11. SQL: LIMIT for limiting the number of results2m
-
12. SQL: UPDATE data in a table6m
-
13. SQL: DELETE data from a table5m
-
14. SQL Wildcards for filtering unknowns5m
-
15. What is a JOIN?14m
-
16. SQL: JOINs and JOIN examples10m
-
17. SQL: GROUP BY for aggregation of data9m
-
18. SQL: ORDER BY for sorting data5m
-
19. SQL: CREATE TABLE12m
-
20. SQL: INSERT INTO for adding data to a table10m
-
21. SQL: SEQUENCE for auto-incrementing fields7m
-
22. SQL: CREATE INDEX and advanced information about indexes12m
-
23. SQL: DROP TABLE for deleting tables and data6m
Advanced PostgreSQL
-
1. SQL: VIEWs and what they are used for19m
-
2. SQL: built-in functions and the HAVING construct12m
-
3. Dates in SQL: an old problem14m
-
4. Other data types in SQL (including JSON in PostgreSQL)11m
-
5. Nested SELECT statements for complex queries9m
-
6. The PostgreSQL SERIAL type3m
Python and PostgreSQL
-
1. Installing psycopg2 on Windows (2018 update)6m
-
2. Installing psycopg2 on Mac4m
-
3. Verifying everything works—don't proceed if it doesn't!3m
-
4. Mac OS X: Fixing psycopg2 'library not loaded' error6m
-
5. What is a virtual environment?8m
-
6. psycopg2 on a virtualenv on Windows (2018 update)4m
-
7. Psycopg2 on a virtualenv on Windows (older versions)5m
-
8. Setting up the app structure, pip, and requirements.txt4m
-
9. Recap on classes and object-oriented programming10m
-
10. Saving to database from Python17m
-
11. Loading from the database from Python16m
-
12. Removing some duplicate code from our app4m
-
13. Connection pooling and why it is important13m
-
14. Creating the ConnectionPool class9m
-
15. Creating the ConnectionFromPool class9m
-
16. Obtaining a cursor from the ConnectionFromPool class8m
-
17. The Database class and selective initialisation19m
-
18. Cleaning up the Database class6m
-
19. End of section review4m
Python Web and APIs
-
1. What is an API?26m
-
2. Making requests in Python12m
-
3. What is OAuth?7m
-
4. Creating a Twitter app4m
-
5. Setting up Twitter login5m
-
6. Getting the OAuth request token15m
-
7. More on the Python debugger—an essential tool5m
-
8. Getting authorization by the user10m
-
9. Getting the OAuth access token6m
-
10. Performing Twitter requests: getting images17m
-
11. Reusing code from the last section to save users11m
-
12. Creating users in our app8m
-
13. Retrieving users in our app14m
-
14. Cleaning up the code—extremely important!19m
-
15. Introduction to Flask and Python Web Development16m
-
16. Adding a Twitter login endpoint11m
-
17. Adding Twitter authorization23m
-
18. Creating the user profile9m
-
19. The Flask before_request decorator9m
-
20. Checking if a user is already logged in3m
-
21. Searching tweets and displaying them8m
-
22. Searching for different things3m
-
23. What is Bootstrap?9m
-
24. Writing our own CSS6m
-
25. Allowing users to perform custom searches7m
-
26. Adding sentiment analysis with another API16m
Introduction to Git and GitHub
-
1. What is Git?5m
-
2. Installing Git on Mac and Windows7m
-
3. Introduction to the UNIX terminal8m
-
4. The VIM text editor, a powerful terminal editor6m
-
5. Dealing with files in the UNIX terminal4m
-
6. What is a Git repository?6m
-
7. git init — create a Git repository4m
-
8. git add and git commit — staging and committing8m
-
9. git log — viewing past commits2m
-
10. Creating a repository on GitHub3m
-
11. git remote — managing remote repositories3m
-
12. Adding your SSH key to GitHub6m
-
13. What is a README file? Introduction to Markdown7m
-
14. git pull — pulling other's changes3m
Advanced Python
-
1. OOP: Inheritance15m
-
2. OOP: Multiple Inheritance in Python5m
-
3. OOP: What is composition?4m
-
4. OOP: What is encapsulation?12m
-
5. Introduction to Exceptions in Python11m
-
6. Creating our own Exceptions7m
-
7. Some of the available built-in Exceptions6m
-
8. Python built-in methods21m
-
9. Assertions in Python8m
-
10. Lambda expressions in Python8m
-
11. More uses of lambda expressions10m
-
12. Lambda expressions in the wild17m
-
13. Introduction to unit testing with unittest20m
Data Structures and Algorithms
-
1. What are data structures?6m
-
2. What is a Linked List?9m
-
3. Introduction to Linked List Assignment10m
-
4. Programming our own Linked List in Python9m
-
5. Creating a Queue6m
-
6. Introduction to Queue Assignment11m
-
7. Programming our own Queue in Python15m
-
8. Creating a Stack4m
-
9. Introduction to Stack Assignment5m
-
10. Programming our own Stack in Python7m
-
11. Introduction to Binary Tree Assignment8m
-
12. Programming our own Binary Tree in Python9m