The Most Complete Python Course
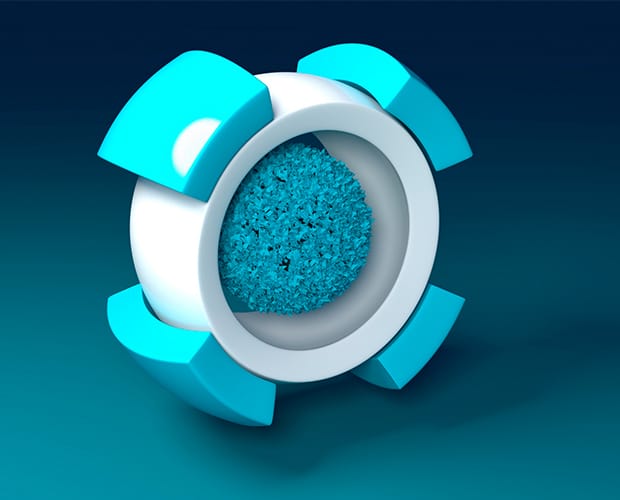
Get ready for your exam by enrolling in our comprehensive training course. This course includes a full set of instructional videos designed to equip you with in-depth knowledge essential for passing the certification exam with flying colors.
$14.99 / $24.99
Introduction
-
1. The Tools You Need1m
-
2. Installing Python 3 and an IDE (Windows, Mac, and Linux)7m
The Basics: Small Program
-
1. Your First Python Code3m
-
2. Your First Python Program10m
The Basics: Data Types
-
1. Variables3m
-
2. Simple Types: Integers, Strings, and Floats3m
-
3. List Types2m
-
4. Type Attributes3m
-
5. How to Find Out What Code You Need5m
-
6. Bonus: Steps of Learning Python1m
-
7. Dictionary Types4m
-
8. Tuple Types2m
-
9. How to Use Datatypes in the Real World?1m
The Basics: Operations with Data Types
-
1. Python Shell and Terminal Tip1m
-
2. More Operations with Lists6m
-
3. Accessing List Items2m
-
4. Accessing List Slices3m
-
5. Accessing Items and Slices with Negative Indexes2m
-
6. Accessing Characters and Slices in Strings2m
-
7. Accessing Items in Dictionaries2m
The Basics: Functions and Conditionals
-
1. Creating Your Own Functions5m
-
2. Print or Return?4m
-
3. Intro to Conditionals1m
-
4. If Conditional Example4m
-
5. Conditional Explained Line by Line3m
-
6. More on Conditionals2m
-
7. Elif Conditionals1m
-
8. White Space4m
The Basics: Processing User Input
-
1. User Input8m
-
2. String Formatting5m
-
3. String Formatting with Multiple Variables4m
Imported Modules
-
1. Builtin Modules6m
-
2. Standard Python Modules9m
-
3. Third-Party Modules6m
-
4. Third-Party Module Example3m
Application 1: Build an Interactive English Dictionary
-
1. Interactive English Dictionary - How The Output Will Look Like4m
-
2. The Data Source5m
-
3. Loading JSON Data4m
-
4. Returning the Definition of a Word3m
-
5. Taking Into Account Bad Words3m
-
6. Implementing Case Sensitivity3m
-
7. Similarity Ratio Between Two Words5m
-
8. Best Matches out of a List of Words6m
-
9. Recommending the Best Match10m
-
10. Confirmation from the User10m
-
11. Optimizing the Final Output8m
Data Analysis with Pandas
-
1. Getting Started with Pandas9m
-
2. Getting Started with Jupyter Notebooks9m
-
3. Loading CSV Files4m
-
4. Loading Excel Files1m
-
5. Loading TXT Files3m
-
6. Set Header Row3m
-
7. Set Column Names1m
-
8. Set Index Column5m
-
9. Indexing and Slicing6m
-
10. Deleting Columns and Rows3m
-
11. Updating and Adding new Columns and Rows8m
-
12. Example: Geocoding Addresses with Pandas and Geopy15m
Numpy
-
1. What is Numpy?8m
-
2. Convert Images to Numpy Arrays6m
-
3. Indexing, Slicing, and Iterating Numpy Arrays4m
-
4. Stacking and Splitting Numpy Arrays6m
Application 2: Create Webmaps with Python and Folium
-
1. Web Map - How The Output Will Look Like1m
-
2. The Basemap12m
-
3. Adding Points8m
-
4. Adding Multiple Points5m
-
5. Adding Points from Files13m
-
6. Popup Windows on Map5m
-
7. Color Points8m
-
8. Solution2m
-
9. GeoJson Data6m
-
10. Adding a GeoJson Polygon Layer3m
-
11. Choropleth Map10m
-
12. Layer Control Panel6m
Application 3: Build a Website Blocker
-
1. Website Blocker - How The Output Will Look Like4m
-
2. Application Architecture4m
-
3. Setting up the Script9m
-
4. Setting up the Infinite Loop11m
-
5. Implementing the First Part12m
-
6. Implementing the Second Part19m
-
7. Scheduling the Python Program on Windows13m
-
8. Scheduling the Python Program on Mac and Linux6m
Application 4: Build a Personal Website with Python and Flask
-
1. Personal Website - How The Output Will Look Like2m
-
2. Your First Website8m
-
3. HTML Templates4m
-
4. Navigation Menu9m
-
5. CSS Styling6m
-
6. Creating a Python Virtual Environment6m
-
7. Deploying the Website to a Live Server22m
-
8. Maintaining the Live Website7m
Graphical User Interfaces with Tkinter
-
1. Introduction to Tkinter3m
-
2. Setting up a GUI with Widgets9m
-
3. Connecting GUI Widgets with Callback Functions10m
Interacting with Databases
-
1. Introduction to "Python with Databases"3m
-
2. Connecting and Inserting Data to SQLite via Python13m
-
3. Selecting, Inserting, Deleting, and Updating SQLite Records7m
-
4. Introduction to PostgreSQL Psycopg29m
-
5. Selecting, Inserting, Deleting, and Updating PostgreSQL Records13m
Application 5: Build a Desktop Database Application
-
1. Desktop Database App - How The Output Will Look Like2m
-
2. User Interface Design6m
-
3. Frontend Interface13m
-
4. Backend24m
-
5. Connecting the Frontend to the Backend, Part 118m
-
6. Connecting the Frontend to the Backend, Part 222m
-
7. Creating a Standalone Executable Version of the Program5m
Object Oriented Programming
-
1. Object Oriented Programming Explained5m
-
2. Turning this Application into OOP Style, Part 113m
-
3. Turning this Application into OOP Style, Part 214m
-
4. Creating a Bank Account Object21m
-
5. Inheritance12m
-
6. OOP Glossary8m
Python for Image and Video Processing with OpenCV
-
1. Introduction2m
-
2. Loading, Displaying, Resizing, and Writing Images14m
-
3. Solution with Explanations4m
-
4. Face Detection20m
-
5. Capturing Video20m
Application 6: Build a Webcam Motion Detector
-
1. Webcam Motion Detector - How The Output Will Look Like2m
-
2. Detecting Webcam Objects30m
-
3. Capturing Motion Time21m
Interactive Data Visualization with Bokeh
-
1. Introduction to Bokeh2m
-
2. Your First Bokeh Plot14m
-
3. Using Bokeh with Pandas5m
-
4. Time-series Plots7m
-
5. More Visualization Examples with Bokeh4m
-
6. Plotting Time Intervals of the Motion Detector14m
-
7. Hover Tool Implementation10m
Webscraping with Python Beautiful Soup
-
1. Section Introduction2m
-
2. The Concept Behind Webscraping5m
-
3. Webscraping Example16m
Application 7: Scrape Real Estate Property Data from the Web
-
1. Scraped Website Data - How The Output Will Look Like2m
-
2. Loading the Webpage in Python7m
-
3. Extracting "div" Tags12m
-
4. Extracting Addresses and Property Details15m
-
5. Extracting Elements without Unique Identifiers12m
-
6. Saving the Extracted Data in CSV Files8m
-
7. Crawling Through Webpages17m
Application 8: Build a Web-based Financial Graph
-
1. Web-based Financial Graph - How The Output Will Look Like2m
-
2. Downloading Datasets with Python12m
-
3. Stock Market Data3m
-
4. Stock Market Data Candlestick Charts6m
-
5. Candlestick Charts with Bokeh Quadrants10m
-
6. Candlestick Charts with Bokeh Rectangles22m
-
7. Candlestick Segments5m
-
8. Stylizing the Chart4m
-
9. The Concept Behind Embedding Bokeh Charts in a Flask Webpage11m
-
10. Embedding the Bokeh Chart in a Webpage16m
-
11. Deploying the Chart Website to a Live Server9m
Application 9: Build a Data Collector Web App with PostGreSQL and Flask
-
1. Data Collector Web App - How The Output Will Look Like3m
-
2. PostGreSQL Database Web App with Flask: Steps6m
-
3. Frontend: HTML Part15m
-
4. Frontend: CSS Part10m
-
5. Backend: Getting User Input18m
-
6. Backend: The PostGreSQL Database Model18m
-
7. Backend: Storing User Data to the Database19m
-
8. Backend: Emailing Database Values Back to the User11m
-
9. Backend: Sending Statistics to Users16m
-
10. Deploying the Web Application to a Live Server30m
-
11. Bonus Lecture: Implementing Download and Upload in your Web App21m