PCAP: Certified Associate in Python Programming
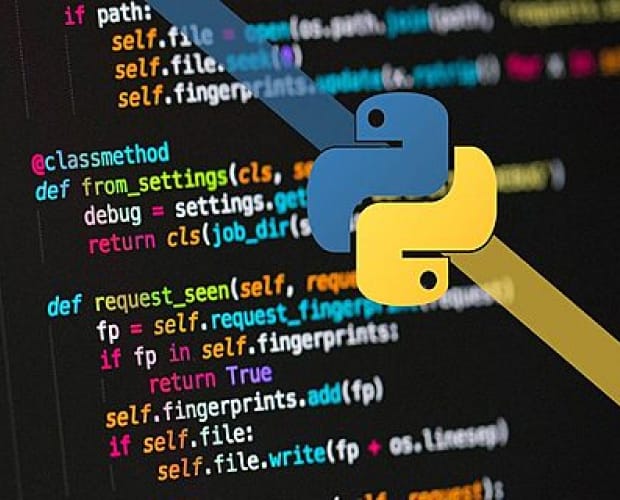
Get ready for your exam by enrolling in our comprehensive training course. This course includes a full set of instructional videos designed to equip you with in-depth knowledge essential for passing the certification exam with flying colors.
$13.74 / $24.99
Introduction
-
1. Configuring Our Dev Environment and Creating Our First Program10m
-
2. Basics of Variables13m
-
3. Dedicated TA Support2m
-
4. Basic Datatypes in Python9m
-
5. Basic Arithmetic in Python4m
-
6. Indexing and Slicing Strings12m
-
7. Basic String Methods13m
-
8. Formatting Strings Using the Format Method5m
-
9. Strings are Immutable3m
-
10. Section 1 Assignments21m
Lists, Tuples and Dictionaries
-
1. Lists in Python17m
-
2. Assignment: List Assignment3m
-
3. Accessing Elements in Nested Lists7m
-
4. Finding Index Positions in Lists and Counting Duplicates4m
-
5. Tuples in Python9m
-
6. Dictionaries in Python16m
-
7. Comparison Operators15m
-
8. Section 2 Assignments18m
Functions and Variable Scope
-
1. Introduction to Creating Functions27m
-
2. *args and **kwargs in Python11m
-
3. Basics of Variable Scope4m
-
4. Scope and Nested Functions9m
-
5. Section 3 Assignments19m
Control Flow
-
1. Control Flow: If & Else Statements13m
-
2. Control Flow: Elif Statements6m
-
3. For Loops20m
-
4. Pass Statement in For Loops5m
-
5. While Loops11m
-
6. Looping and Unpacking with Dictionaries and Tuples13m
-
7. Range, Enumerate, and Zip Functions11m
-
8. More Handy Functions and the Random Package15m
-
9. Accepting Input From a User10m
-
10. Section 4 Assignments: Part 118m
-
11. Section 4 Assignments: Part 216m
-
12. Section 4 Assignments: Part 321m
-
13. Section 4 Assignments: Advanced Assignment21m
Modules, Packages and Object Oriented Programming in Python
-
1. Revisiting the Difference between Methods and Functions8m
-
2. Classes and Objects11m
-
3. Classes Attributes vs Object Attributes20m
-
4. Calling Python Code That is Saved in Another File4m
-
5. Inheritance and Polymorphism18m
-
6. Abstract Classes and Methods12m
-
7. Practical Application of OOP16m
-
8. Double Under (Dunder) Methods6m
-
9. Python Script Files11m
-
10. Python Modules and Using Code from Other Files7m
-
11. Python Packages17m
-
12. Understanding the if __name__ == __main__ Syntax11m
-
13. Section 5 Assignment10m
File IO and Exception Handling in Python
-
1. Exception Handling10m
-
2. File IO26m
-
3. File IO with Exception Handling20m
-
4. OS Module16m
-
5. Traversing Directories Using the OS Module10m
-
6. OS Module Continued11m
-
7. argv Command Line Arguments and the re Module9m
-
8. Section 6 Assignment21m