NodeJS: Complete Developer Guide to Learn and Understand
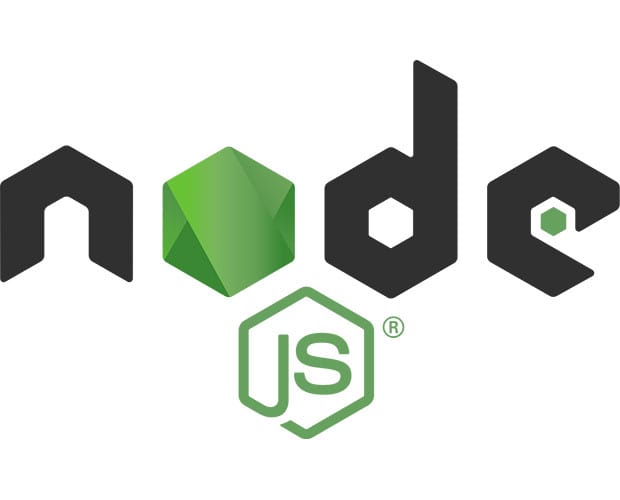
Get ready for your exam by enrolling in our comprehensive training course. This course includes a full set of instructional videos designed to equip you with in-depth knowledge essential for passing the certification exam with flying colors.
$14.99 / $24.99
Introduction and Setup
-
1. Introduction and the Goal of this Course4m 33s
-
2. Big Words and NodeJS1m 13s
-
3. Conceptual Aside: The Command Line Interface9m 49s
V8: The Javascript Engine
-
1. Conceptual Aside: Processors, Machine Language, and C++10m 7s
-
2. Javascript Aside: Javascript Engines and The ECMAScript Specification4m 1s
-
3. V8 Under the Hood5m 57s
-
4. Adding Features to Javascript15m 4s
The Node Core
-
1. Conceptual Aside: Servers and Clients6m 45s
-
2. What Does Javascript Need to Manage a Server?2m 18s
-
3. The C++ Core5m 51s
-
4. The Javascript Core3m 4s
-
5. Let's Install and Run Some Javascript in Node16m 47s
Modules, Exports, and Require
-
1. Conceptual Aside: Modules2m 21s
-
2. Javascript Aside: First-Class Functions and Function Expressions12m 3s
-
3. Let's Build a Module10m 54s
-
4. Javascript Aside: Objects and Object Literals6m 55s
-
5. Javascript Aside: Prototypal Inheritance and Function Constructors11m 38s
-
6. Javascript Aside: By Reference and By Value5m 44s
-
7. Javascript Aside: Immediately Invoked Function Expressions (IIFEs)7m 42s
-
8. How Do Node Modules Really Work?: module.exports and require17m 33s
-
9. Javascript Aside: JSON1m 40s
-
10. More on require11m 39s
-
11. Module Patterns19m 12s
-
12. exports vs module.exports10m 2s
-
13. Requiring Native (Core) Modules6m 51s
-
14. Modules and ES62m 36s
-
15. Web Server Checklist1m 21s
Events and the Event Emitter
-
1. Conceptual Aside: Events5m 24s
-
2. Javascript Aside: Object Properties, First Class Functions, and Arrays5m 8s
-
3. The Node Event Emitter - Part 113m 58s
-
4. The Node Event Emitter - Part 211m 58s
-
5. Javascript Aside: Object.create and Prototypes6m 18s
-
6. Inheriting From the Event Emitter14m 40s
-
7. Javascript Aside: Node, ES6, and Template Literals7m 55s
-
8. Javascript Aside: .call and .apply3m 40s
-
9. Inheriting From the Event Emitter - Part 29m 43s
-
10. Javascript Aside: ES6 Classes8m 43s
-
11. Inheriting From the Event Emitter - Part 36m
Asynchronous Code, libuv, The Event Loop, Streams, Files, and more…
-
1. Javascript Aside: Javascript is Synchronous2m 38s
-
2. Conceptual Aside: Callbacks1m 29s
-
3. libuv, The Event Loop, and Non-Blocking Asynchronous Execution11m 39s
-
4. Conceptual Aside: Streams and Buffers4m 31s
-
5. Conceptual Aside: Binary Data, Character Sets, and Encodings11m 9s
-
6. Buffers6m 48s
-
7. ES6 Typed Arrays4m 52s
-
8. Javascript Aside: Callbacks4m 3s
-
9. Files and fs15m 52s
-
10. Streams18m 14s
-
11. Conceptual Aside: Pipes2m 14s
-
12. Pipes15m 51s
-
13. Web Server Checklist2m 37s
HTTP and being a Web Server
-
1. Conceptual Aside: TCP/IP7m 55s
-
2. Conceptual Aside: Addresses and Ports3m 11s
-
3. Conceptual Aside: HTTP6m 6s
-
4. http_parser6m 56s
-
5. Let's Build a Web Server in Node17m 26s
-
6. Outputting HTML and Templates11m 43s
-
7. Streams and Performance5m 11s
-
8. Conceptual Aside: APIs and Endpoints2m 37s
-
9. Outputting JSON6m 39s
-
10. Routing11m 2s
-
11. Web Server Checklist2m 24s
NPM: the Node Package Manager
-
1. Conceptual Aside: Packages and Package Managers3m 7s
-
2. Conceptual Aside: Semantic Versioning (semver)4m 46s
-
3. npm and the npm registry: Other People's Code4m 59s
-
4. init, nodemon, and package.json13m 2s
-
5. init, nodemon, and package.json - Part 215m 17s
-
6. Using Other People's Code1m 43s
Express
-
1. Installing Express and Making it Easier to Build a Web Server16m 1s
-
2. Routes4m 32s
-
3. Static Files and Middleware14m 58s
-
4. Templates and Template Engines15m 54s
-
5. Querystring and Post Parameters18m 21s
-
6. RESTful APIs and JSON5m 21s
-
7. Structuring an App13m 45s
Javascript, JSON, and Databases
-
1. Conceptual Aside: Relational Databases and SQL3m 48s
-
2. Node and MySQL9m 28s
-
3. Conceptual Aside: NoSQL and Documents3m 12s
-
4. MongoDB and Mongoose10m 50s
-
5. Web Server Checklist1m 7s
The MEAN stack
-
1. MongoDB, Express, AngularJS, and NodeJS6m 58s
-
2. AngularJS: Managing the Client10m 32s
-
3. AngularJS: Managing the Client (Part 2)8m 9s
-
4. AngularJS: Managing the Client (Part 3)19m 17s
-
5. Conceptual Aside: Angular 1, Angular 2, React, and more…3m 6s
-
6. Working with The Full Stack (and being a Full Stack Developer) - Part 111m 59s
Let's Build an App! (in record time)
-
1. NodeTodo: Software Requirements2m 20s
-
2. Initial Setup3m 38s
-
3. Setting up Mongo and Mongoose9m 9s
-
4. Adding Seed Data9m 31s
-
5. Creating our API13m 33s
-
6. Testing our API16m 17s
-
7. Adding a Front-End in Angular 2 (Part 1)23m 22s