Golang Comprehensive Course
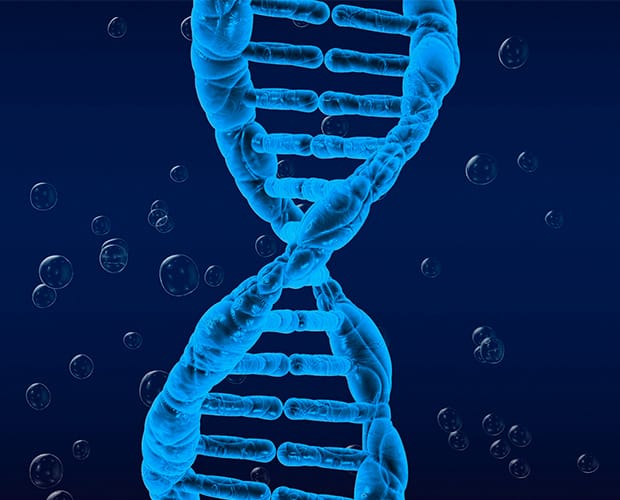
Get ready for your exam by enrolling in our comprehensive training course. This course includes a full set of instructional videos designed to equip you with in-depth knowledge essential for passing the certification exam with flying colors.
$14.99 / $24.99
Getting Started
-
1. Environment Setup3m
-
2. VSCode Installation3m
-
3. Go Support in VSCode3m
A Simple Start
-
1. Boring Ol' Hello World3m
-
2. Five Important Questions6m
-
3. Go Packages6m
-
4. Import Statements4m
-
5. File Organization4m
Deeper Into Go
-
1. Project Overview3m
-
2. New Project Folder2m
-
3. Variable Declarations11m
-
4. Functions and Return Types8m
-
5. Slices and For Loops11m
-
6. OO Approach vs Go Approach5m
-
7. Custom Type Declarations7m
-
8. Receiver Functions6m
-
9. Creating a New Deck11m
-
10. Slice Range Syntax6m
-
11. Multiple Return Values8m
-
12. Byte Slices7m
-
13. Deck to String7m
-
14. Joining a Slice of Strings7m
-
15. Saving Data to the Hard Drive7m
-
16. Reading From the Hard Drive11m
-
17. Error Handling8m
-
18. Shuffling a Deck10m
-
19. Random Number Generation11m
-
20. Testing With Go4m
-
21. Writing Useful Tests12m
-
22. Asserting Elements in a Slice4m
-
23. Testing File IO10m
-
24. Project Review6m
Organizing Data With Structs
-
1. Structs in Go5m
-
2. Defining Structs4m
-
3. Declaring Structs5m
-
4. Updating Struct Values6m
-
5. Embedding Structs7m
-
6. Structs with Receiver Functions7m
-
7. Pass By Value6m
-
8. Structs with Pointers3m
-
9. Pointer Operations10m
-
10. Pointer Shortcut6m
-
11. Gotchas With Pointers4m
-
12. Reference vs Value Types8m
Maps
-
1. What's a Map?6m
-
2. Manipulating Maps5m
-
3. Iterating Over Maps5m
-
4. Differences Between Maps and Structs6m
Interfaces
-
1. Purpose of Interfaces9m
-
2. Problems Without Interfaces10m
-
3. Interfaces in Practice9m
-
4. Rules of Interfaces8m
-
5. Extra Interface Notes7m
-
6. The HTTP Package8m
-
7. Reading the Docs6m
-
8. More Interface Syntax3m
-
9. Interface Review2m
-
10. The Reader Interface8m
-
11. More on the Reader Interface7m
-
12. Working with the Read Function6m
-
13. The Writer Interface4m
-
14. The io.Copy Function5m
-
15. The Implementation of io.Copy5m
-
16. A Custom Writer8m
Channels and Go Routines
-
1. Website Status Checker5m
-
2. Printing Site Status5m
-
3. Serial Link Checking3m
-
4. Go Routines7m
-
5. Theory of Go Routines9m
-
6. Channels6m
-
7. Channel Implementation9m
-
8. Blocking Channels10m
-
9. Receiving Messages4m
-
10. Repeating Routines7m
-
11. Alternative Loop Syntax4m
-
12. Sleeping a Routine6m
-
13. Function Literals5m
-
14. Channels Gotcha!11m