Building Frontend Applications with Vue JS
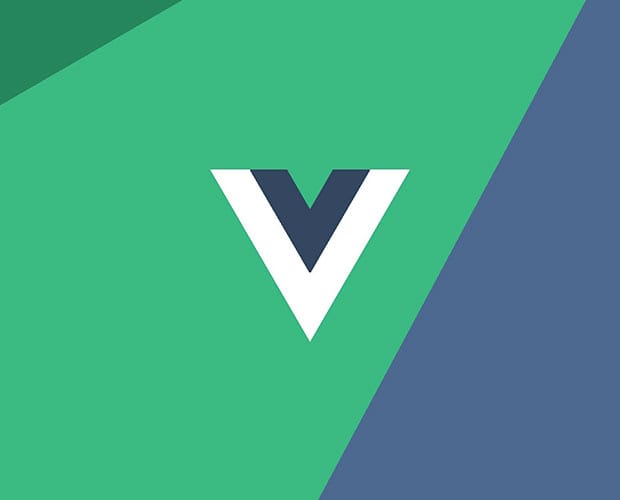
Get ready for your exam by enrolling in our comprehensive training course. This course includes a full set of instructional videos designed to equip you with in-depth knowledge essential for passing the certification exam with flying colors.
$14.99 / $24.99
Getting Started
-
1. Course Introduction3m 9s
-
2. Let's Create our First VueJS Application4m 45s
-
3. Extending the VueJS Application4m 12s
-
4. Course Structure3m 44s
-
5. Take Advantage of all Course Resources!1m 56s
-
6. Setup VueJS Locally2m 3s
Using VueJS to Interact with the DOM
-
1. Module Introduction45s
-
2. Understanding VueJS Templates2m 35s
-
3. How the VueJS Template Syntax and Instance Work Together2m 42s
-
4. Accessing Data in the Vue Instance1m 49s
-
5. Binding to Attributes2m 43s
-
6. Understanding and Using Directives1m 27s
-
7. Disable Re-Rendering with v-once1m 41s
-
8. How to Output Raw HTML2m 43s
-
9. Listening to Events1m 50s
-
10. Getting Event Data from the Event Object2m 36s
-
11. Passing your own Arguments with Events1m 53s
-
12. Modifying an Event - with Event Modifiers3m 23s
-
13. Listening to Keyboard Events2m 3s
-
14. Writing JavaScript Code in the Templates3m 19s
-
15. Using Two-Way-Binding1m 49s
-
16. Reacting to Changes with Computed Properties9m 32s
-
17. An Alternative to Computed Properties: Watching for Changes3m 53s
-
18. Saving Time with Shorthands1m 26s
-
19. Dynamic Styling with CSS Classes - Basics4m 22s
-
20. Dynamic Styling with CSS Classes - Using Objects1m 36s
-
21. Dynamic Styling with CSS Classes - Using Names3m 27s
-
22. Setting Styles Dynamically (without CSS Classes)3m 15s
-
23. Styling Elements with the Array Syntax1m 14s
-
24. Module Wrap Up44s
Using Conditionals and Rendering Lists
-
1. Module Introduction49s
-
2. Conditional Rendering with v-if3m 38s
-
3. Using an Alternative v-if Syntax1m 36s
-
4. Don't Detach it with v-show1m 43s
-
5. Rendering Lists with v-for2m 26s
-
6. Getting the Current Index1m 51s
-
7. Using an Alternative v-for Syntax1m 10s
-
8. Looping through Objects4m 44s
-
9. Looping through a List of Numbers57s
-
10. Keeping Track of Elements when using v-for4m 8s
-
11. Module Wrap Up51s
First Course Project - The Monster Slayer
-
1. Introduction & Challenge2m 54s
-
2. Setting up the Course Project2m 37s
-
3. Creating the Vue Instance and Styling the Healthbars5m 16s
-
4. Showing the Player Controls Conditionally2m 2s
-
5. Implementing a "Start Game" Method1m 53s
-
6. Implementing a "Attack" Method8m 3s
-
7. Write better Code - Time for Refactoring!4m 52s
-
8. Implementing a "Special Attack"2m 11s
-
9. Implementing a "Heal" Method2m 21s
-
10. Finishing the Action Buttons59s
-
11. Creating an Action Log2m 41s
-
12. Printing the Log (v-for)2m 20s
-
13. Finishing the Log1m 57s
-
14. Styling the Log Conditionally1m 44s
-
15. Wrap Up1m 28s
Understanding the VueJS Instance
-
1. Module Introduction1m 4s
-
2. Some Basics about the VueJS Instance2m 58s
-
3. Using Multiple Vue Instances2m 52s
-
4. Accessing the Vue Instance from Outside3m 29s
-
5. How VueJS manages your Data and Methods4m 1s
-
6. A Closer Look at $el and $data4m 55s
-
7. Placing $refs and Using them on your Templates5m 48s
-
8. Where to learn more about the Vue API58s
-
9. Mounting a Template6m 42s
-
10. Using Components4m 56s
-
11. Limitations of some Templates2m 35s
-
12. How VueJS Updates the DOM3m 25s
-
13. The VueJS Instance Lifecycle3m 12s
-
14. The VueJS Instance Lifecycle in Practice5m 39s
-
15. Module Wrap Up55s
Moving to a "Real" Development Workflow with Webpack and Vue CLI
-
1. Module Introduction1m 34s
-
2. Why do we need a Development Server?2m 47s
-
3. What does "Development Workflow" mean?3m 51s
-
4. Using the Vue CLI to create Projects2m 35s
-
5. Installing the Vue CLI and Creating a new Project4m 18s
-
6. An Overview over the Webpack Template Folder Structure3m 40s
-
7. Understanding ".vue" Files6m 46s
-
8. Understanding the Object in the Vue File1m 11s
-
9. How to Build your App for Production49s
-
10. Module Wrap Up1m 11s
An Introduction to Components
-
1. Module Introduction1m 4s
-
2. An Introduction to Components4m 56s
-
3. Storing Data in Components with the Data Method4m 4s
-
4. Registering Components Locally and Globally2m 50s
-
5. The "Root Component" in the App.vue File3m 21s
-
6. Creating a Component4m 24s
-
7. Using Components5m 32s
-
8. Moving to a Better Folder Structure2m 43s
-
9. How to Name your Component Tags (Selectors)4m 31s
-
10. Scoping Component Styles5m 5s
-
11. Module Wrap Up50s
Communicating between Components
-
1. Module Introduction46s
-
2. Communication Problems2m 52s
-
3. Using Props for Parent => Child Communication3m 10s
-
4. Naming "props"1m 10s
-
5. Using "props" in the Child Component1m 28s
-
6. Validating "props"4m 57s
-
7. Using Custom Events for Child => Parent Communication5m 55s
-
8. Understanding Unidirectional Data Flow1m 7s
-
9. Communicating with Callback Functions2m 23s
-
10. Communication between Sibling Components6m 25s
-
11. Using an Event Bus for Communication5m 32s
-
12. Centralizing Code in an Event Bus2m 14s
-
13. Wrap Up41s
Advanced Component Usage
-
1. Module Introduction38s
-
2. Setting up the Module Project3m
-
3. Passing Content - The Suboptimal Solution2m 19s
-
4. Passing Content with Slots42s
-
5. How Slot Content gets Compiled and Styled3m 3s
-
6. Using Multiple Slots (Named Slots)2m 50s
-
7. Default Slots and Slot Defaults2m 1s
-
8. A Summary on Slots45s
-
9. Switching Multiple Components with Dynamic Components5m 31s
-
10. Understanding Dynamic Component Behavior2m 7s
-
11. Keeping Dynamic Components Alive58s
-
12. Dynamic Component Lifecycle Hooks1m 18s
-
13. Wrap Up1m 11s
Second Course Project - Wonderful Quotes
-
1. Module Introduction1m 36s
-
2. Setting up the Project1m 22s
-
3. Initializing the Application1m 28s
-
4. Creating the Application Components4m 4s
-
5. Passing Data with Props and Slots2m 13s
-
6. Allowing Users to Create Quotes with a NewQuote Component4m 8s
-
7. Adding Quotes with Custom Events3m 34s
-
8. Adding a Info Box1m 6s
-
9. Allowing for Quote Deletion3m 40s
-
10. Controlling Quotes with a Progress Bar3m 52s
-
11. Finishing Touches and State Management2m 13s
Handling User Input with Forms
-
1. Module Introduction54s
-
2. A Basic 'input' Form Binding3m 46s
-
3. Grouping Data and Pre-Populating Inputs2m 42s
-
4. Modifying User Input with Input Modifiers2m 58s
-
5. Binding 'textarea' and Saving Line Breaks2m 53s
-
6. Using Checkboxes and Saving Data in Arrays4m 5s
-
7. Using Radio Buttons2m 30s
-
8. Handling Dropdowns with 'select' and 'option'5m 9s
-
9. What v-model does and How to Create a Custom Control2m 49s
-
10. Creating a Custom Control (Input)5m 13s
-
11. Submitting a Form2m 8s
-
12. Wrap Up43s
Using and Creating Directives
-
1. Module Introduction53s
-
2. Understanding Directives3m 34s
-
3. How Directives Work - Hook Functions2m 28s
-
4. Creating a Simple Directive1m 22s
-
5. Passing Values to Custom Directives1m 8s
-
6. Passing Arguments to Custom Directives2m 40s
-
7. Modifying a Custom Directive with Modifiers2m 25s
-
8. Custom Directives - A Summary28s
-
9. Registering Directives Locally1m 37s
-
10. Using Multiple Modifiers4m 27s
-
11. Passing more Complex Values to Directives2m 13s
-
12. Wrap Up44s
Improving your App with Filters and Mixins
-
1. Module Introduction45s
-
2. Creating a Local Filter4m 32s
-
3. Global Filters and How to Chain Multiple Filters1m 45s
-
4. An (often-times better) Alternative to Filters: Computed Properties5m 15s
-
5. Understanding Mixins2m 23s
-
6. Creating and Using Mixins2m 25s
-
7. How Mixins get Merged2m 58s
-
8. Creating a Global Mixin (Special Case!)3m 26s
-
9. Mixins and Scope2m 33s
-
10. Wrap Up1m 56s
Adding Animations and Transitions
-
1. Module Introduction1m 8s
-
2. Understanding Transitions1m 1s
-
3. Preparing Code to use Transitions3m 11s
-
4. Setting Up a Transition3m 9s
-
5. Assigning CSS Classes for Transitions2m 27s
-
6. Creating a "Fade" Transition with the CSS Transition Property3m 21s
-
7. Creating a "Slide" Transition with the CSS Animation Property3m 59s
-
8. Mixing Transition and Animation Properties3m 31s
-
9. Animating v-if and v-show33s
-
10. Setting Up an Initial (on-load) Animation1m 30s
-
11. Using Different CSS Class Names4m 1s
-
12. Using Dynamic Names and Attributes2m 33s
-
13. Transitioning between Multiple Elements (Theory)34s
-
14. Transitioning between Multiple Elements (Practice)4m 42s
-
15. Listening to Transition Event Hooks2m 6s
-
16. Understanding JavaScript Animations8m 3s
-
17. Excluding CSS from your Animation1m 28s
-
18. Creating an Animation in JavaScript6m 18s
-
19. Animating Dynamic Components5m 57s
-
20. Animating Lists with 'transition-group'44s
-
21. Using 'transition-group' - Preparations4m 20s
-
22. Using 'transition-group' to Animate a List6m 12s
-
23. Understanding the App1m 15s
-
24. Creating the App12m 4s
-
25. Adding Animations6m 38s
-
26. Wrap Up1m 3s
Connecting to Servers via Http - Using vue-resource
-
1. Module Introduction1m 42s
-
2. Accessing Http via vue-resource - Setup4m
-
3. Creating an Application and Setting Up a Server (Firebase)5m 24s
-
4. POSTing Data to a Server (Sending a POST Request)6m 10s
-
5. GETting and Transforming Data (Sending a GET Request)6m 54s
-
6. Configuring vue-resource Globally2m 48s
-
7. Intercepting Requests3m 34s
-
8. Intercepting Responses2m 51s
-
9. Where the "resource" in vue-resource Comes From4m 42s
-
10. Creating Custom Resources2m 39s
-
11. Resources vs "Normal" Http Requests28s
-
12. Understanding Template URLs5m 6s
-
13. Wrap Up1m 42s
Routing in a VueJS Application
-
1. Module Introduction1m 38s
-
2. Setting up the VueJS Router (vue-router)2m 16s
-
3. Setting Up and Loading Routes6m 43s
-
4. Understanding Routing Modes (Hash vs History)4m 14s
-
5. Navigating with Router Links3m 45s
-
6. Where am I? - Styling Active Links3m 20s
-
7. Navigating from Code (Imperative Navigation)2m 42s
-
8. Setting Up Route Parameters1m 59s
-
9. Fetching and Using Route Parameters2m 3s
-
10. Reacting to Changes in Route Parameters3m 4s
-
11. Setting Up Child Routes (Nested Routes)4m 39s
-
12. Navigating to Nested Routes3m 9s
-
13. Making Router Links more Dynamic2m 4s
-
14. A Better Way of Creating Links - With Named Routes3m 21s
-
15. Using Query Parameters2m 54s
-
16. Multiple Router Views (Named Router Views)3m 27s
-
17. Redirecting2m 13s
-
18. Setting Up "Catch All" Routes / Wildcards1m 6s
-
19. Animating Route Transitions2m 34s
-
20. Passing the Hash Fragment3m 28s
-
21. Controlling the Scroll Behavior3m 21s
-
22. Protecting Routes with Guards1m 13s
-
23. Using the "beforeEnter" Guard7m 51s
-
24. Using the "beforeLeave" Guard3m 31s
-
25. Loading Routes Lazily7m 8s
-
26. Wrap Up1m 2s
Better State Management with Vuex
-
1. Module Introduction1m 12s
-
2. Why a Different State Management May Be Needed2m 25s
-
3. Understanding "Centralized State"1m 32s
-
4. Using the Centralized State6m 49s
-
5. Why a Centralized State Alone Won't Fix It2m 41s
-
6. Understanding Getters48s
-
7. Using Getters2m 40s
-
8. Mapping Getters to Properties6m 57s
-
9. Understanding Mutations1m 14s
-
10. Using Mutations4m 51s
-
11. Why Mutations have to run Synchronously1m 34s
-
12. How Actions improve Mutations1m 32s
-
13. Using Actions4m 59s
-
14. Mapping Actions to Methods4m 58s
-
15. A Summary of Vuex4m 14s
-
16. Two-Way-Binding (v-model) and Vuex5m 56s
-
17. Improving Folder Structures1m 1s
-
18. Modularizing the State Management4m 56s
-
19. Using Separate Files4m 18s
-
20. Using Namespaces to Avoid Naming Problems6m 44s
-
21. Wrap Up50s
Final Project - The Stock Trader
-
1. Project Introduction3m 11s
-
2. Project Setup and Planning2m 45s
-
3. Creating the First Components2m 55s
-
4. Setup Project Routes4m 42s
-
5. Adding a Header and Navigation6m 44s
-
6. Planning the Next Steps1m 22s
-
7. Creating Stocks Components6m 31s
-
8. Adding a "Buy" Button5m 57s
-
9. Setting up the Vuex State Management10m 21s
-
10. Adding a Portfolio Module to Vuex10m 2s
-
11. Working on the Portfolio Stocks6m 51s
-
12. Connecting the Portfolio with Vuex2m 21s
-
13. Time to fix some Errors2m
-
14. Displaying the Funds3m 41s
-
15. Adding some Order Checks5m 41s
-
16. Making Funds Look Nicer with Filters1m 48s
-
17. Ending the Day - Randomizing Stocks5m 30s
-
18. Animating the Route Transitions3m 14s
-
19. Saving & Fetching Data - Adding a Dropdown2m 17s
-
20. Setting up vue-resource and Firebase2m 16s
-
21. Saving Data (PUT Request)2m 51s
-
22. Fetching Data (GET Request)5m 4s
-
23. Testing and Bug Fixes2m 50s
-
24. Project Wrap Up1m 22s
-
25. Bonus: Debugging Vuex with Vue Developer Tools1m 59s
Deploying a VueJS Application
-
1. Module Introduction1m 12s
-
2. Preparing for Deployment1m 45s
-
3. Deploying the App (Example: AWS S3)5m 57s
Course Roundup
-
1. Courses Roundup59s
All Course Exercises ("Time to Practice")
-
1. Time to Practice (1) - Outputting Data to Templates (Problem)1m 59s
-
2. Time to Practice (1) - Outputting Data to Templates (Solution)6m 12s
-
3. Time to Practice (2) - Events (Problem)1m 42s
-
4. Time to Practice (2) - Events (Solution)4m 54s
-
5. Time to Practice (3) - Reactive Properties (Problem)2m 29s
-
6. Time to Practice (3) - Reactive Properties (Solution)5m 47s
-
7. Time to Practice (4) - Styling (Problem)3m 49s
-
8. Time to Practice (4) - Styling (Solution)18m 17s
-
9. Time to Practice (5) - Conditionals and Lists (Problem)1m 40s
-
10. Time to Practice (5) - Conditionals and Lists (Solution)9m 38s
-
11. Time to Practice (6) - Components (Problem)1m 45s
-
12. Time to Practice (6) - Components (Solution)2m 12s
-
13. Time to Practice (7) - Component Communication (Problem)3m 6s
-
14. Time to Practice (7) - Component Communication (Solution)10m 17s
-
15. Time to Practice (8) - Slots and Dynamic Components (Problem)1m 27s
-
16. Time to Practice (8) - Slots and Dynamic Components (Solution)3m
-
17. Time to Practice (9) - Forms (Problem)1m 30s
-
18. Time to Practice (9) - Forms (Solution)15m 17s
-
19. Time to Practice (10) - Directives (Problem)54s
-
20. Time to Practice (10) - Directives (Solution)5m 55s
-
21. Time to Practice (11) - Filters and Mixins (Problem)1m 8s
-
22. Time to Practice (11) - Filters and Mixins (Solution)8m 12s