The Complete Developer Course: ES6 Javascript
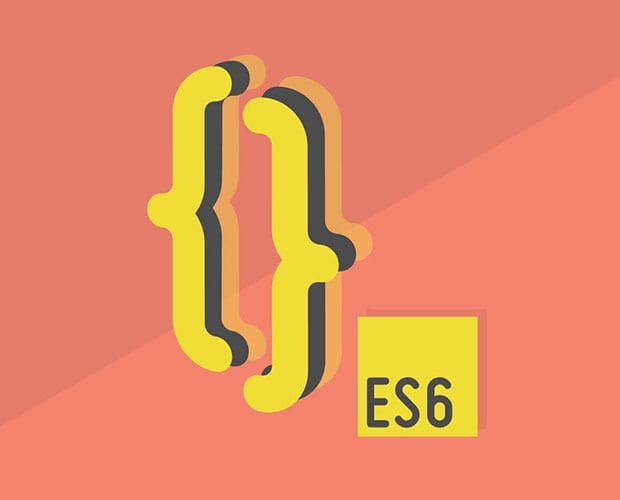
Get ready for your exam by enrolling in our comprehensive training course. This course includes a full set of instructional videos designed to equip you with in-depth knowledge essential for passing the certification exam with flying colors.
$14.99 / $24.99
Before We Get Started
-
1. How to Take This Course5m 3s
-
2. ES6 vs ES20156m
The 'forEach' Helper
-
1. Array Helper Methods - The Easiest Way to Write Better Code3m 9s
-
2. The forEach Helper7m 21s
-
3. forEach Continued4m 23s
-
4. Why Use forEach?3m 3s
The 'map' Helper
-
1. The Map Helper6m 35s
-
2. Map Helper Continued2m 13s
-
3. Where Map Is Used2m 22s
The 'filter' Helper
-
1. Selecting Needed Data with Filter9m 11s
-
2. More on Filtering3m 30s
-
3. Choosing When to Filter3m 22s
The 'find' Helper
-
1. Querying For Records with Find6m 46s
-
2. Find Continued4m 9s
-
3. Using Find to Search for Users2m 31s
The 'every' and 'some' Helper
-
1. A Little Every and a Lot of Some10m 2s
-
2. More on Every and Some4m 47s
-
3. Every and Some Syntax2m 24s
-
4. Every and Some in Practice5m 34s
The 'reduce' Helper
-
1. Condensing Lists with Reduce5m 46s
-
2. A Touch More of Reduce4m 42s
-
3. Ace Your Next Interview with Reduce9m 37s
Const/Let
-
1. Variable Declarations with Const and Let6m 38s
-
2. What Const and Let Solve8m 14s
Template Strings
-
1. Template Strings4m 45s
-
2. When to Reach for Template Strings7m 31s
Arrow Functions
-
1. Fat Arrow Functions6m 14s
-
2. Advanced Use of Arrow Functions6m 55s
-
3. When to Use Arrow Functions6m 38s
-
4. When to Use Arrow Functions Continued3m 19s
Enhanced Object Literals
-
1. Enhanced Object Literals9m 35s
-
2. Wondering When to Use Enhanced Literals?4m 31s
Default Function Arguments
-
1. Specifying Default Function Arguments4m 49s
-
2. Use Cases of Defaulting Arguments4m 57s
Rest and Spread Operator
-
1. Capturing Arguments with Rest and Spread4m 34s
-
2. The Rest on Rest and Spread7m 52s
-
3. Look to Use Rest and Spread in This Case4m 59s
Destructuring
-
1. Goldmine of ES6: Destructuring5m 39s
-
2. Destructuring Arguments Object4m 47s
-
3. Destructuring Arrays4m 46s
-
4. Destructuring Arrays and Objects *At the Same Time*5m 44s
-
5. So...When to Use Destructuring?7m 46s
-
6. More on When to Use Destructuring6m 26s
Classes
-
1. Introduction to Classes3m 53s
-
2. Prototypal Inheritance4m 26s
-
3. Refactoring with Classes4m 52s
-
4. Extending Behavior of Classes9m
-
5. When to Use Classes3m 57s
Generators
-
1. One Quick Thing: For...Of Loops3m 3s
-
2. Introduction to Generators5m 56s
-
3. Generators With a Short Story13m 9s
-
4. Another Step in Our Generator Story4m 51s
-
5. The Big Reveal on ES6 Generators4m 32s
-
6. A Practical Use of ES6 Generators3m 47s
-
7. Delegation of Generators5m 26s
-
8. Delegation of Generators Continued7m 8s
-
9. Symbol.Iterator with Generators6m 46s
-
10. Complexities of Symbol.Iterator4m 57s
-
11. Generators with Recursion6m 45s
-
12. More on Generators and Recursion6m 44s
Promises and Fetch
-
1. Code Execution in Javascript4m 44s
-
2. Terminology of Promises3m 54s
-
3. Creating Promises7m
-
4. Then and Catch5m 49s
-
5. Async Code with Promises3m 13s
-
6. Ajax Requests with Fetch5m 24s
-
7. Shortcomings of Fetch5m 57s