Fundamentals of Data Structures and Algorithms: Fundamentals of Data Structures & Algorithms
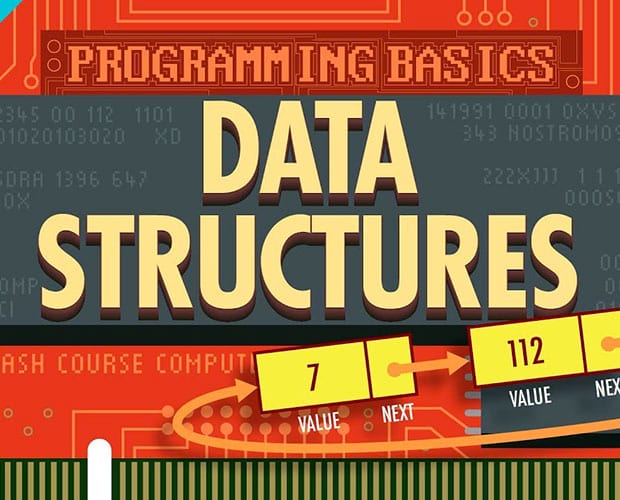
Get ready for your exam by enrolling in our comprehensive training course. This course includes a full set of instructional videos designed to equip you with in-depth knowledge essential for passing the certification exam with flying colors.
$14.99 / $24.99
Introduction to Algorithms
-
1. Introduction1m 8s
-
2. Euclid's algorithm4m 49s
-
3. Bubble Sort algorithm2m 52s
-
4. Why study data structures & algorithms3m 10s
-
5. Correctness of an algorithm1m 35s
Analysis of Algorithms
-
1. Introduction3m 20s
-
2. How to calculate the time complexity2m 52s
-
3. The RAM model of computation2m 7s
-
4. Time complexity of Bubble sort algorithm3m 25s
-
5. Pseudo code : Bubble sort algorithm3m 2s
-
6. The Big O notation3m 26s
-
7. Using Big O notation : Examples4m 41s
-
8. Comparison of running times4m 2s
Basic Sorting and Search Algorithms
-
1. Selection Sort2m 48s
-
2. Selection Sort : Pseudocode2m 34s
-
3. Introduction to Insertion Sort1m 56s
-
4. Applying Insertion Sort algorithm to cue balls2m 8s
-
5. Insertion Sort: Pseudocode2m 38s
-
6. O(n²) sorting algorithms - Comparison2m
-
7. Stable Vs Unstable Sorts3m 46s
-
8. Searching elements in an un ordered array3m 16s
-
9. Searching elements in an ORDERED array2m 33s
-
10. Searching elements in an ORDERED array - contd.5m 48s
-
11. Inserting and Deleting items in an ORDERED array2m 8s
-
12. Sorting any type of object1m 33s
Linked Lists
-
1. What is a Linked List?3m 21s
-
2. Implementing a Linked List in Java56s
-
3. Inserting a new Node5m 25s
-
4. Length of a Linked List2m 11s
-
5. Deleting the head node2m 11s
-
6. Searching for an Item3m 11s
-
7. Doubly Ended Lists3m 6s
-
8. Inserting data in a sorted Linked List4m 38s
-
9. Doubly Linked List6m 28s
-
10. Insertion Sort revisited10m 32s
Stacks and Queues
-
1. Stacks2m 41s
-
2. Abstract Data Types37s
-
3. Implementing Stacks using Arrays3m 21s
-
4. Queues2m 32s
-
5. Queues using Arrays5m 29s
-
6. Double Ended Queues1m 58s
-
7. Double Ended Queues using Arrays4m 20s
Recursion
-
1. Introduction4m 33s
-
2. Understanding Recursion3m 4s
-
3. Tail recursion2m 8s
-
4. Tower of Hanoi8m 25s
-
5. Tower of Hanoi - Implementation2m 58s
-
6. Merge Sort4m 9s
-
7. Merge Sort - Pseudocode4m 24s
-
8. Merge Step - Pseudocode4m 32s
-
9. Time Complexity of Merge Sort2m 52s
Binary Search Trees
-
1. The Tree Data structure3m 41s
-
2. Binary Trees3m 34s
-
3. Binary Search Trees2m 1s
-
4. Finding an item in a Binary Search Tree2m 24s
-
5. Implementing the find method3m 2s
-
6. Inserting an item in a Binary Search Tree3m 34s
-
7. Deleting an Item : Case 16m 6s
-
8. Deleting an Item - Case 22m 58s
-
9. Deleting an Item - Case 33m 44s
-
10. Deleting an Item - Soft Delete1m 40s
-
11. Finding smallest & largest values2m 33s
-
12. Tree Traversal : In Order3m 19s
-
13. Tree Traversal : Pre Order1m 58s
-
14. Tree Traversal : Post Order56s
-
15. Unbalanced Trees Vs Balanced Trees2m 16s
-
16. Height of a Binary Tree1m 34s
-
17. Time Complexity of Operations on Binary Search Trees2m 16s
More Sorting Algorithms
-
1. Introduction1m 27s
-
2. QuickSort4m 54s
-
3. QuickSort: The partition step2m 21s
-
4. Shell Sort5m 27s
-
5. Shell Sort: Example3m 28s
-
6. Counting Sort4m 50s
-
7. Radix Sort2m 27s
-
8. Bucket Sort3m 12s
Heaps
-
1. Introduction4m 6s
-
2. Deleting the root1m 54s
-
3. Inserting an item in a heap1m 59s
-
4. Heaps as Priority Queues2m 30s
-
5. Representing heaps using Arrays1m 55s
-
6. Heap Sort2m 30s
-
7. Building a heap4m 7s
Hashtables
-
1. Introduction2m 41s
-
2. Direct Access Tables2m 4s
-
3. Hashing1m 37s
-
4. Resolving collisions through chaining4m 16s
-
5. The Hash function4m 16s
-
6. Open Addressing to resolve collisions2m 58s
-
7. Strategies for Open Addressing3m 19s
-
8. Time Complexity: Open Addressing3m 20s
-
9. Conclusion59s