Complete React Native and Hooks Course
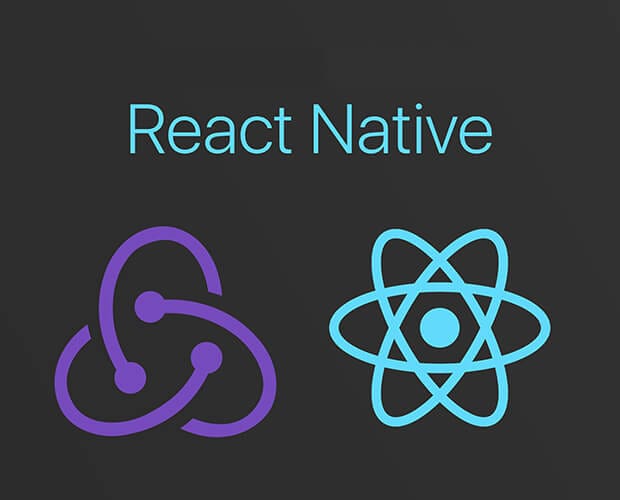
Get ready for your exam by enrolling in our comprehensive training course. This course includes a full set of instructional videos designed to equip you with in-depth knowledge essential for passing the certification exam with flying colors.
$14.99 / $24.99
Getting Started
-
1. How to Get Help1m
-
2. Course Overview3m
-
3. App Setup9m
-
4. Making Changes4m
Working with Content
-
1. Overview of React Components9m
-
2. Showing a Custom Component4m
-
3. Common Questions and Answers7m
-
4. Rules of JSX6m
-
5. One Common Error2m
-
6. JSX Exercise Overview2m
-
7. JSX Solution3m
List Building - With Style!
-
1. Building Lists2m
-
2. The FlatList Element2m
-
3. Rendering a FlatList5m
-
4. Why a Key Property?4m
-
5. Solving the Key Issue5m
-
6. A Few Props Around FlatList5m
-
7. Exercise Overview1m
-
8. Exercise Solution2m
Navigating Users Between Screens
-
1. Button Types4m
-
2. Buttons in Action5m
-
3. Touchable Opacity in Action4m
-
4. Navigating with React Navigation7m
-
5. Destructuring Props4m
Building Reusable Components
-
1. Component Reuse with Props4m
-
2. Exercise Solution2m
-
3. Parent-Child Relationships5m
-
4. Communicating from Parent to Child6m
-
5. Showing Images4m
-
6. Passing Images as Props4m
-
7. Exercise Outline1m
State Management in React Components
-
1. State in Components6m
-
2. Screen Boilerplate3m
-
3. State in Action10m
-
4. Notes on State7m
-
5. App Overview5m
-
6. Generating Random Colors4m
-
7. Adding Random Colors5m
-
8. Showing Colors with a FlatList4m
-
10. Reusable Color Adjusters6m
-
11. Coordinating State6m
-
12. Passing Callbacks to Children6m
-
13. Tying State Values Together7m
-
14. Validating State Changes8m
-
15. Reusable State Updates8m
-
16. Introduction to Reducers9m
-
17. Creating a Reducer15m
-
18. Applying State with a Reducer9m
-
19. Restoring Validation7m
-
20. Community Convention in Reducers7m
-
21. Exercise Outline1m
-
22. Exercise Solution11m
-
23. Handling Text Input3m
-
24. Showing a Text Input3m
-
25. Two Important Props4m
-
26. Weird Things with Text and State6m
-
27. Updating State5m
How to Handle Screen Layout
-
1. Layout with React Native4m
-
2. Basics of Box Object Model6m
-
3. AlignItems with Flex5m
-
4. Flex Direction4m
-
5. Justify Content3m
-
6. Flex Values5m
-
7. Align Self on Children2m
-
8. The Position Property3m
-
9. Top, Bottom, Left, Right3m
-
10. Absolute Fill Objects3m
-
11. Applying Layout Systems2m
-
12. Exercise Overview1m
-
13. Exercise Solution6m
Putting It All Together - Restaurant Search App
-
1. App Overview2m
-
2. Project Generation3m
-
3. Yelp Signup5m
-
4. Yelp Walkthrough4m
-
5. React Navigation3m
-
6. Assembling a Navigator7m
-
7. Architecture Approach3m
-
8. Starting the SearchBar4m
-
9. Displaying Icons6m
-
10. Search Bar Styling4m
-
11. A Touch More Styling4m
-
12. Managing State7m
-
13. Detecting Editing Completion4m
Using Outside API's
-
1. Configuring Axios7m
-
2. Making the Request11m
-
3. Error Handling8m
-
4. Running an Initial Search6m
Making Hooks Reusable
-
1. The UseEffect Hook4m
-
2. Extracting Hook Logic8m
-
3. Showing Search Results6m
-
4. Grouping Results7m
-
5. FlatList Rendering4m
Navigation with Parameters
-
1. Showing a Single Result4m
-
2. Showing Additional Info6m
-
3. A Few More Styling Issues7m
-
4. Hiding Scroll Bars3m
-
5. Constraining View Elements3m
-
6. Empty Elements3m
-
7. Spacing on the Search Bar2m
-
8. Reminder on Navigation5m
-
9. Navigating from a Child Component3m
-
10. The WithNavigation Helper3m
-
11. Communicating Between Screens4m
-
12. Fetching a Single Restaurant6m
-
13. Showing a List of Images4m
-
14. One Last Fix2m
Advanced State Management with Context
-
1. App Overview3m
-
2. Issues with Data6m
-
3. Initial Setup5m
-
4. Wrapping the Navigator3m
-
5. Introduction to Context2m
-
6. Adding Context6m
-
7. Moving Data with Context6m
-
8. Rendering a List of Posts4m
-
9. Adding State in Context8m
-
10. It Works!4m
-
11. Opportunity for Improvement2m
-
12. Updating with UseReducer10m
-
13. Automating Context Creation12m
-
14. More Automatic Context Creation9m
-
15. A Bit of Styling7m
-
16. Deleting Posts4m
-
17. Updating the Reducer6m
-
18. Navigation on Tap5m
-
19. Retrieving Single Posts5m
-
20. Adding a Creation Screen2m
-
21. Header Navigation5m
-
22. Displaying a Form7m
-
23. Saving a New Post5m
-
24. Navigation on Save6m
-
25. The Edit Icon Link5m
-
26. Communicating Info to Edit6m
-
27. Initializing State from Context6m
-
28. Extracting Form Logic9m
-
29. Customizing OnSubmit4m
-
30. Initial Form Values5m
-
31. Default Props2m
-
32. Editing Action Function5m
-
33. Editing in a Reducer4m
-
34. Navigating Backwards4m
Data API Sync
-
1. Outside Data API2m
-
2. Issues with Servers + React Native3m
-
3. JSON Server and Ngrok Setup7m
-
4. JSON Server REST Conventions4m
-
5. Making a Request10m
-
6. Remote Fetch of Posts6m
-
7. Creating Posts with Post Requests4m
-
8. Refetching on Navigate6m
-
9. Deleting a Post3m
-
10. Editing Posts2m
-
11. App Wrapup3m
Building a Custom Express API
-
1. App Overview5m
-
2. Dependencies Setup2m
-
3. The Basics of Express4m
-
4. MongoDB Setup4m
-
5. Connecting to MongoDB7m
-
6. Nodemon for Automatic Restarts1m
-
7. Understanding the Signup Process6m
-
8. Using Postman3m
-
9. Handling JSON Data5m
-
10. Defining a User Schema7m
-
11. Creating and Saving a User5m
-
12. Error Handling6m
-
13. JSON Web Tokens7m
-
14. Creating a JWT3m
-
15. Wiring Up JSON Web Tokens13m
-
16. Understanding Password Hashing8m
-
17. Salting and Hashing9m
-
18. The Signin Route7m
-
19. Testing Signup and Signin3m
-
20. Defining Tracks7m
-
21. Listing Tracks8m
-
22. Creating Tracks10m
In-App Authentication
-
1. Server Setup8m
-
2. Navigation Design11m
-
3. A LOT of Boilerplate7m
-
4. Navigator Hookup8m
-
5. Testing the Navigation Flow6m
-
6. React Native Elements5m
-
7. Helper Styling Components9m
-
8. Styling Odds and Ends7m
-
9. Input Props5m
-
10. The Auth Context11m
-
11. What's the Context Doing?8m
-
12. Axios Setup6m
-
13. Making an API Request10m
-
14. Handling Errored Requests8m
-
15. Async Storage4m
-
16. Storing the Token7m
-
17. Navigation From Outside of React13m
-
18. Oops, Typo1m
-
19. Navigation to Signin4m
-
20. Extracting Form Logic10m
-
21. Last Bit of Extractin5m
-
22. Creating a NavLink7m
-
23. Real Component Reuse!5m
-
24. Wiring Up Signin8m
-
25. Clearing Error Messages11m
-
26. Automatic Signin6m
-
27. Empty Screens While Resolving Auth7m
-
28. Signing Out a User7m
-
29. Safe Area Views2m
-
30. Working on Track Create5m
-
31. Showing a Map5m
-
32. Drawing a Series of Points5m
-
33. Notes on Location4m
-
34. Requesting Location Permissions7m
-
35. Resetting Permissions4m
-
36. How to Test Location?4m
-
37. Faking the Users Location6m
-
38. Reading a Location4m
-
39. Bugginess with Location3m
-
40. Location Architecture4m
-
41. Location Context4m
-
42. Live Location Updates14m
-
43. Drawing a Position Indicator4m
-
44. Extracting Logic to a Hook8m
-
45. Disabling Location Tracking4m
-
46. Automatic Disables8m
-
47. Building a Track Form4m
-
48. Updates to Location Context9m
-
49. Track Form Wire Up6m
-
50. Buggy UseEffects9m
-
51. Understanding Stale References13m
-
52. Kind of Fixed7m
-
53. The UseCallback Hook7m
-
54. Cleaning Up After Ourselves6m
-
55. Avoiding Stale References7m
-
56. Tracking While Recording2m
-
57. Bring Back the Polyline4m
-
58. What Manages Tracks8m
-
59. Coordination Between Contexts8m
-
60. Automatic Authentication10m
-
61. Form Reset and Navigation7m
-
62. Fetching Created Tracks2m
-
63. Listing All Tracks7m
-
64. Navigating to a Saved Track5m
-
65. Showing Track Details5m
-
66. Fixing Odds and Ends9m
[LEGACY] Dive Right In!
-
1. Introduction - Course Roadmap2m
-
2. Roadmap to the First App2m
[LEGACY] Got OSX? Go Here.
-
1. OSX Installation6m
-
2. More on OSX Installation5m
-
3. Running in the Simulator2m
[LEGACY] Got Windows? Go Here.
-
1. Windows Setup of React Native7m
-
2. Android Studio and React Native CLI Installation5m
-
3. Emulator Creation and System Variables15m
[LEGACY] [Optional] ESLint Setup
-
1. ESLint Setup and Overview9m
-
2. ESLint Setup with Atom6m
-
3. ESLint Setup with Sublime Text 38m
-
4. ESLint Setup with VSCode4m
[LEGACY] Onwards!
-
1. Project Directory Walkthrough2m
-
2. Getting Content on the Screen3m
-
3. React vs React Native3m
-
4. Creating a Component with JSX6m
-
5. Registering a Component4m
-
6. Destructuring Imports5m
-
7. Application Outline5m
-
8. The Header Component8m
-
9. Consuming File Exports7m
[LEGACY] Making Great Looking Apps
-
1. Styling with React Native5m
-
2. More on Styling Components5m
-
3. Introduction to Flexbox5m
-
4. Header Styling4m
-
5. Making the Header Reusable7m
[LEGACY] HTTP Requests with React Native
-
1. Class Based Components7m
-
2. Lifecycle Methods7m
-
3. Network Requests8m
-
4. Component Level State9m
-
5. More on Component Level State7m
-
6. Rendering a List of Components6m
-
7. Displaying Individual Albums10m
-
8. Fantastic Reusable Components - The Card5m
-
9. Styling a Card6m
-
10. Passing Components as Props4m
-
11. Dividing Cards into Sections8m
[LEGACY] Handling Component Layout
-
1. Mastering Layout with Flexbox5m
-
2. Positioning of Elements on Mobile5m
-
3. More on Styling4m
-
4. Images with React Native8m
-
5. Displaying Album Artwork9m
-
6. Making Content Scrollable5m
-
7. Handling User Input with Buttons8m
-
8. Styling of Buttons with UX Considerations5m
-
9. Responding to User Input7m
-
10. Linking Between Mobile Apps4m
-
11. Setting Button Text by Props2m
-
12. App Wrapup3m
[LEGACY] Authentication with Firebase
-
1. Auth App Introduction5m
-
2. A Common Root Component4m
-
3. Copying Reusable Components11m
-
4. What is Firebase?5m
-
5. Firebase Client Setup5m
-
6. Login Form Scaffolding6m
-
7. Handling User Inputs3m
-
8. More on Handling User Inputs3m
-
9. How to Create Controlled Components5m
-
10. Making Text Inputs From Scratch4m
-
11. A Focus on Passing Props5m
-
12. Making the Input Pretty6m
-
13. Wrapping up Inputs7m
-
14. Password Inputs9m
[LEGACY] Processing Authentication Credentials
-
1. Logging a User In6m
-
2. Error Handling7m
-
3. More on Authentication Flow8m
-
4. Creating an Activity Spinner5m
-
5. Conditional Rendering of JSX7m
-
6. Clearing the Form Spinner8m
-
7. Handling Authentication Events6m
-
8. More on Conditional Rendering7m
-
9. Logging a User Out and Wrapup6m
[LEGACY] Digging Deeper with Redux
-
1. App Mockup and Approach4m
-
2. The Basics of Redux9m
-
3. More on Redux8m
-
4. Redux is Hard!12m
-
5. Application Boilerplate3m
-
6. More on Redux Boilerplate8m
[LEGACY] Back to React
-
1. Rendering the Header6m
-
2. Reducer and State Design7m
-
3. Library List of Data8m
-
4. The Connect Function10m
-
5. MapStateToProps with Connect8m
-
6. A Quick Review and Breather6m
[LEGACY] Rendering Lists the Right Way
-
1. The Theory of ListView7m
-
2. ListView in Practice4m
-
3. Rendering a Single Row2m
-
4. Styling the List7m
-
5. Creating the Selection Reducer5m
-
6. Introducing Action Creators6m
-
7. Calling Action Creators9m
-
8. Adding a Touchable7m
-
9. Rules of Reducers5m
-
10. Expanding a Row5m
-
11. Moving Logic Out of Components6m
-
12. Animations7m
-
13. Wrapup5m
[LEGACY] Not Done Yet...
-
1. Overview of Our Next App5m
-
2. App Challenges5m
-
3. Just a Touch More Setup6m
-
4. More on Boilerplate Setup7m
[LEGACY] Handling Data in React vs Redux
-
1. Login Form in a Redux World7m
-
2. Rebuilding the Login Form6m
-
3. Handling Form Updates with Action Creators9m
-
4. Wiring up Action Creators8m
-
5. Typed Actions9m
[LEGACY] Don't Mutate that State
-
1. Synchronous vs Asynchronous Action Creators8m
-
2. Introduction to Redux Thunk6m
-
3. Redux Thunk in Practice6m
-
4. Redux Thunk in Practice Continued8m
-
5. Making LoginUser More Robust3m
-
6. Creating User Accounts7m
-
7. Showing Error Messages7m
-
8. A Firebase Gotcha7m
-
9. Showing a Spinner on Loading12m
[LEGACY] Navigating Users Around
-
1. Dealing with Navigation8m
-
2. Navigation in the Router5m
-
3. Addressing Styling Issues5m
-
4. Displaying Multiple Scenes6m
-
5. Navigating Between Routes4m
-
6. Grouping Scenes with Buckets7m
-
7. Navigation Bar Buttons3m
-
8. Navigating to the Employee Creation Form6m
-
9. Building the Employee Creation Form5m
-
10. Employee Form Actions7m
-
11. Handling Form Updates at the Reducer Level6m
-
12. Dynamic Property Updates9m
-
13. The Picker Component8m
-
14. Pickers and Style Overrides7m
[LEGACY] Firebase as a Data Store
-
1. Firebase JSON Schema7m
-
2. Data Security in Firebase6m
-
3. Creating Records with Firebase6m
-
4. Default Form Values6m
-
5. Successful Data Save to Firebase8m
-
6. Resetting Form Properties10m
-
7. Fetching Data from Firebase8m
-
8. Storing Data by ID9m
-
9. Dynamic DataSource Building8m
-
10. Transforming Objects to Arrays7m
-
11. List Building in Employee List6m
[LEGACY] Code Reuse - Edit vs Create
-
1. Reusing the Employee Form7m
-
2. Create vs Edit Forms7m
-
3. Reusable Forms9m
-
4. A Standalone Employee Edit Form3m
-
5. Initializing Forms from State9m
-
6. Updating Firebase Records7m
-
7. Clearing Form Attributes5m
-
8. Texting Employees5m
-
9. Modals as a Reusable Component7m
-
10. The Modal Component Continued8m
-
11. Styling the Modal9m
-
12. Employee Delete Action Creator9m
-
13. Wiring up Employee Delete4m