Detailed Walkthroughs on Advanced React and Redux concepts
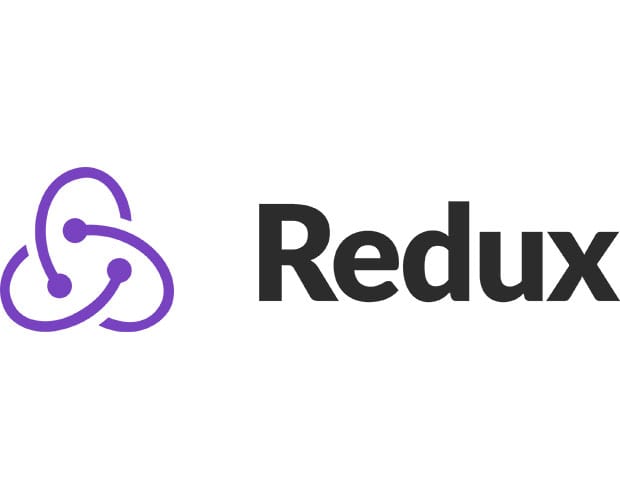
Get ready for your exam by enrolling in our comprehensive training course. This course includes a full set of instructional videos designed to equip you with in-depth knowledge essential for passing the certification exam with flying colors.
$14.99 / $24.99
Welcome! Let's Get Started!
-
1. Introduction1m 46s
Testing
-
1. Project Setup3m 29s
-
2. Core Testing - Describe, It, Expect9m 39s
-
3. A First Spec12m 42s
-
4. Test Reporting4m 5s
-
5. Feature Mockups6m 9s
-
6. Test Structure Setup6m 55s
-
7. Comment Box Tests8m 19s
-
8. Testing Class Names7m 50s
-
9. Using beforeEach to Condense Tests6m 22s
-
10. Expecting Child Elements5m 13s
-
11. Simulating Events7m 24s
-
12. Testing Controlled Components7m 23s
-
13. Form Submit Event7m 13s
-
14. Stub Comment List6m
-
15. Expectations on Content7m 43s
-
16. Assertions with Lists5m 42s
-
17. Testing Action Creators9m 34s
-
18. Action Creator Shortcuts4m 47s
-
19. TDD Comments Reducer12m 37s
-
20. Spec Failures After Code Change3m 36s
-
21. App Review3m 7s
Test Environment Setup
-
1. Purpose of Chai and Mocha5m 19s
-
2. Test Helper From Scratch2m 43s
-
3. JSDom Setup7m 14s
-
4. More JSDom Setup5m 28s
-
5. TestUtils Library7m 53s
-
6. Defining RenderComponent7m 52s
-
7. Finishing RenderComponent Helper5m 12s
-
8. Simulate Helper7m 9s
-
9. Test Helper Review3m 8s
Higher Order Components
-
1. What is a Higher Order Component?3m 6s
-
2. Connect and Provider5m 49s
-
3. Authentication HOC Overview4m 19s
-
4. Header Setup6m 54s
-
5. React Router Setup7m 36s
-
6. Authentication Reducer7m 5s
-
7. Action Creator Hookup8m 4s
-
8. Authentication Higher Order Component4m 57s
-
9. HOC Scaffold Code9m 9s
-
10. Nesting Higher Order Components4m 48s
-
11. Accessing React Router on Context5m 30s
-
12. Class Level Properties2m 12s
-
13. Handling HOC Edge Cases5m 3s
-
14. Higher Order Components Review3m 5s
Middleware
-
1. Middleware Overview3m 26s
-
2. App Building Plan3m 50s
-
3. Users Reducer4m 14s
-
4. Static Users Action Creator2m 15s
-
5. Rendering a List of Users7m 32s
-
6. CSS Cleanup4m 20s
-
7. Pains Without Middleware7m 15s
-
8. Middleware Stack4m 43s
-
9. Middleware Internals7m 55s
-
10. Handling Unrelated Actions5m 17s
-
11. Handling Promises8m 5s
-
12. Middleware Review6m 50s
Server Setup - Authentication
-
1. Introduction to Authentication4m 32s
-
2. Cookies vs Tokens4m 57s
-
3. Scalable Architecture4m 49s
-
4. Server Setup3m 45s
-
5. More Server Setup5m 41s
-
6. Express Middleware6m 29s
-
7. Express Route Handler6m 9s
-
8. Mongoose Models10m 22s
-
9. MongoDB Setup6m 29s
-
10. Inspecting the Database6m 19s
-
11. Authentication Controller4m 57s
-
12. Searching for Users7m 57s
-
13. Creating User Records6m 31s
-
14. Encrypting Passwords with Bcrypt8m 12s
-
15. Salting a Password6m 16s
-
16. JWT Overview4m 58s
-
17. Creating a JWT7m 13s
-
18. Installing Passport5m 51s
-
19. Passport Strategies8m 55s
-
20. Using Strategies with Passport4m 2s
-
21. Making an Authenticated Request5m 55s
-
22. Signing in with Local Strategy5m 37s
-
23. Purpose of Local Strategy4m 17s
-
24. Bcrypt Full Circle5m 8s
-
25. Protecting Signin Route4m 12s
-
26. Signing Users In4m 4s
-
27. Server Review2m 39s
Client Side Authentication
-
1. Client Setup2m 28s
-
2. App Architecture5m 48s
-
3. Component and State Design7m 30s
-
4. Header Component4m 17s
-
5. Scaffolding the Signin Form11m 3s
-
6. Adding Signin Form5m 58s
-
7. Action Creator with Many Responsibilities8m 24s
-
8. Introducing Redux Thunk7m 10s
-
9. Signin Action Creator10m 38s
-
10. CORS In a Nutshell9m 13s
-
11. Serverside Solution for CORS6m 17s
-
12. Programmatic Navigation7m 11s
-
13. Updating Auth State6m 29s
-
14. Breather and Review5m 39s
-
15. LocalStorage and JWT6m 39s
-
16. Auth Error Messaging4m 36s
-
17. Displaying Errors4m 40s
-
18. Header Logic10m 29s
-
19. Signout Component5m 32s
-
20. Signout Action Creator4m 45s
-
21. Signup Component4m 12s
-
22. Signup Form Scaffolding8m 2s
-
23. Redux Form Validation4m 30s
-
24. Implementing Validation Logic9m 28s
-
25. More On Validation5m 40s
-
26. Signup Action Creator7m 11s
-
27. Finish Up Signup7m 11s
-
28. Securing Individual Routes10m 21s
-
29. Root IndexRoute2m 34s
-
30. Automatically Authenticating Users8m 1s
-
31. Making Authenticated API Requests3m 29s
-
32. Handling Data from Authenticated Requests5m 13s
-
33. Authentication Wrapup3m 29s